By default, the header length limit is 65536 which is set in HKEY_LOCAL_MACHINE\System\CurrentControlSet\Services\HTTP\Parameters registry. I tested it both on my local machine and Azure Web App. Following is the code which I tested.
On server side I use
public class HomeController : Controller
{
public ActionResult Index()
{
return Content(Request.Headers["Authorization"]);
}
}
On client side, I use
static async void SendRequest()
{
HttpClient client = new HttpClient();
string token = "";
for (int i = 0; i < 2050; i++)
{
token = token + "0";
}
client.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Bearer", token);
HttpResponseMessage message = await client.GetAsync("http://xxx.azurewebsites.net/");
string content = await message.Content.ReadAsStringAsync();
Console.WriteLine(content);
}
I can get the Authorization parameter back.
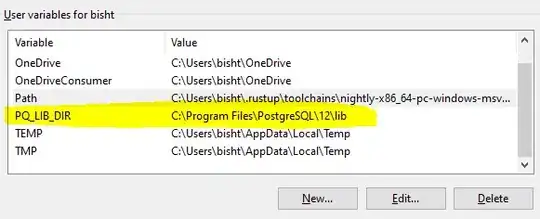
Using trial and error I figured out that header value limit must be around 2048
Another way which would modified the limit is the headerLimits config section. We could add length limit for specific header using this config section.
If I add following configuration to web.config. The request from my client was blocked and I got following error.
<system.webServer>
<security>
<requestFiltering>
<requestLimits>
<headerLimits >
<add header="Authorization" sizeLimit="2048" />
</headerLimits>
</requestLimits>
</requestFiltering>
</security>
</system.webServer>
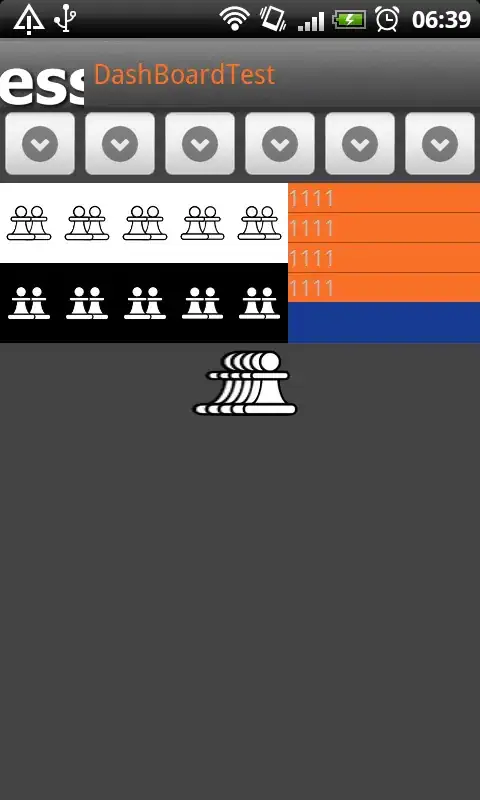
If I increase the sizeLimit to meet the length of request Authorization header, for example 2058. The request will be executed OK.
So please check whether you have modified the headerLimits config section in your web.config file. If yes, It will block your request if the length of this header is larger than the limit value. To solve it, we can increase the value of sizeLimit to modify the limit of Authorization header.