Swing has no such animal as a textbox -- do you mean JTextField? If so,...
- You would create a JTextField by simply doing just that --
new JTextField()
- Then would add it to your GUI by calling
add(...)
on an appropriate container such as a JPanel.
- You would then read the text by simply calling
getText()
on it, and the JTextField tutorials will explain all of this.
- You will also want to read the tutorial on the layout managers since the container that accepts the JTextField must have a layout that can accommodate the new component.
- You will also want to call
revalidate()
and repaint()
on the container after adding or removing components so that the containers layout manager will know to update its layout and repaint itself.
This is just a general gist of what needs to be done. If you need more specific advice, please tell us the details of your problem, what you've tried so far, and what has worked or failed.
Edit
You ask:
But how do I do this so that the textField is a "pop up" rather than an addition to the current container. I have it so that it adds to the current container...but that is not what I need.
- The easiest way to do this is by displaying a JOptionPane.showInputDialog(...).
For example:
// myGui is the currently displayed GUI
String foo = JOptionPane.showInputDialog(myGui, "Message", "Title",
JOptionPane.PLAIN_MESSAGE);
System.out.println(foo);
This would look like so:

- A more complex way of doing this is to create a GUI and display it in a JOptionPane, and the query the GUI for its contents.
For example:
JTextField fooField = new JTextField(15);
JTextField barField = new JTextField(15);
JPanel moreComplexPanel = new JPanel(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
gbc.insets = new Insets(5, 5, 5, 5);
gbc.weightx = 1.0;
gbc.weighty = 1.0;
gbc.anchor = GridBagConstraints.WEST;
moreComplexPanel.add(new JLabel("Foo:"), gbc);
gbc.gridx = 1;
gbc.anchor = GridBagConstraints.EAST;
moreComplexPanel.add(fooField, gbc);
gbc.gridx = 0;
gbc.gridy = 1;
gbc.anchor = GridBagConstraints.WEST;
moreComplexPanel.add(new JLabel("Bar:"), gbc);
gbc.gridx = 1;
gbc.anchor = GridBagConstraints.EAST;
moreComplexPanel.add(barField, gbc);
int result = JOptionPane.showConfirmDialog(myGui, moreComplexPanel,
"Foobars Forever", JOptionPane.OK_CANCEL_OPTION,
JOptionPane.PLAIN_MESSAGE);
if (result == JOptionPane.OK_OPTION) {
System.out.println("foo = " + fooField.getText());;
System.out.println("bar = " + barField.getText());;
}
Which would look like:
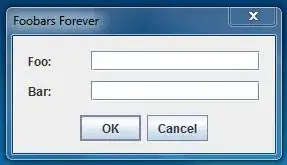