Let's look at this scenario :
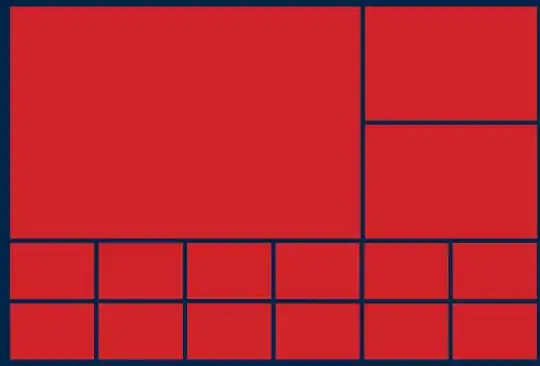
In your view, you have a button. When it's clicked, you want to play the video directly.
In order, to do so, you open the webview as a modal view of your view :
[self presentModalViewController:videoWebView animated:NO];
For your webview, you should use Youtube API to integrate and autoplay the video. See the proposed working example here : https://stackoverflow.com/a/15538968
You'll see that the video is launched in a modal view of your webview view. One way to detect when the video is dismissed (when the "done" button has been clicked) is to use the viewDidAppear
on your webview view class. In this method you will then dismiss the webview view too but...when this view is launched at first, you don't want to dismiss it. You can add a boolean property to avoid that.
- (void)viewDidAppear:(BOOL)animated {
[super viewDidAppear:animated];
if (_videoLaunched) {
[self dismissModalViewControllerAnimated:YES];
}
}
In the viewDidLoad
method, set this property to NO and in the webViewDidFinishLoad
method (delegate method of webview) set it to YES.
I think it answers one part of your question. Concerning the detection of the end of the video you have to modify you YT_Player.html
file to listen to state changes.
ytPlayer = new YT.Player('media_area', {height: '100%', width: '100%', videoId: 'SbPFDcspRBA',
events: {'onReady': onPlayerReady, 'onStateChange': onPlayerStateChange}
function onPlayerStateChange(e) {
var result = JSON.parse(event.data);
if (result.info == 0) { // Video end
window.location = "videomessage://end";
}
}
});
You will then catch the event in your webview view and dismiss it like this :
-(BOOL)webView:(UIWebView *)webView shouldStartLoadWithRequest:(NSURLRequest *)request navigationType:(UIWebViewNavigationType)navigationType {
NSURL *url = request.URL;
if ([[url scheme] isEqualToString:@"videomessage"]) {
[self dismissModalViewControllerAnimated:YES];
return YES;
}
return YES;
}