Building up on what @ja72 suggested, it is very easy to parse data from the link which he mentioned. I am not too good with C# so here is a vb.net code which you can convert to C#
Having said that, there are many ways you can look at this problem from C#
WAY 1
Navigate to the URL at runtime and parse the values using the below code.
Disadvantage:
1) You have to have an internet connection
2) This process is slow
WAY 2
Create a separate program to navigate to the URL and parse the values using the below code.
Use the below code to generate the output to a text file or a csv file and embed the file in your resource so that you can work with it anytime you want
I would suggest WAY 2 but the decision is finally yours. :)
CODE
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
TextBox1.Clear()
GetFormulas()
MessageBox.Show ("Done!")
End Sub
Sub GetFormulas()
Dim wc As New Net.WebClient
'~~> first get links
Dim mainPage As String = wc.DownloadString("http://office.microsoft.com/client/helppreview.aspx?AssetId=HV805551279990&lcid=1033&NS=EXCEL.DEV&Version=12&pid=CH080555125")
Dim doc As mshtml.IHTMLDocument2 = New mshtml.HTMLDocument
doc.write (mainPage)
doc.close()
Dim table As mshtml.IHTMLElement = DirectCast(doc.getElementById("vstable"), mshtml.IHTMLElement2).getElementsByTagName("table")(0)
Dim links As mshtml.IHTMLElementCollection = table.getElementsByTagName("A")
For Each link In links
Dim childPage As String = wc.DownloadString(link.getAttribute("href"))
Dim doc2 As mshtml.IHTMLDocument2 = New mshtml.HTMLDocument
doc2.write (childPage)
doc2.close()
Dim div2 As mshtml.IHTMLElement2 = doc2.getElementById("m_article")
For Each elem As mshtml.IHTMLElement In div2.getElementsByTagName("P")
If elem.getAttribute("className") = "signature" Then
Dim formulaString As String = elem.innerText
TextBox1.AppendText (link.innerText & vbTab & formulaString & vbCrLf)
End If
Next
Next
End Sub
End Class
SNAPSHOT
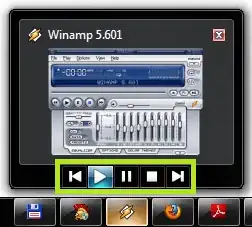
NOTE: The above is just an example on how to scrape the above link given by ja72. In case you plan to go with any other link then you will have to change the code accordingly. Also note that the formulas mentioned in the above link are for Excel 2007 onwards. For Excel 2003 you will have to go in for another link. I have not included a STOP
button in the above example so once the program starts running, it cannot be stopped till it finishes. I am sure that you can add one more button to terminate the extraction.