Use a JToggleButton
as shown in Swing JToolbarButton pressing.

In your use-case, the green unselected image will simply be either a fully transparent image, or an image that is the desired BG color.
More specific example:
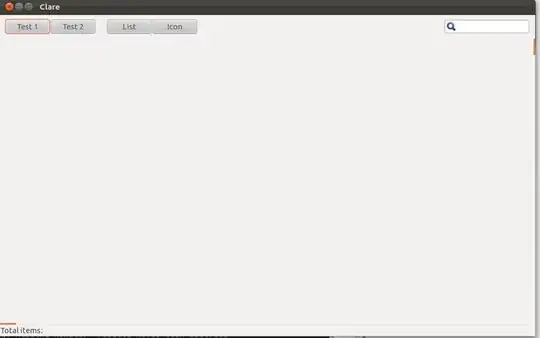
import java.awt.*;
import java.awt.image.*;
import javax.swing.*;
class ToggleImage {
public static JToggleButton getButton(
Image selected,
Image unselected) {
JToggleButton b = new JToggleButton();
b.setSelectedIcon(new ImageIcon(selected));
b.setIcon(new ImageIcon(unselected));
b.setBorderPainted(false);
b.setContentAreaFilled(false);
b.setFocusPainted(false);
b.setMargin(new Insets(0,0,0,0));
return b;
}
public static Image getImage(boolean hasSquare) {
int size = 60;
BufferedImage bi = new BufferedImage(
size,size,BufferedImage.TYPE_INT_ARGB);
Graphics2D g = bi.createGraphics();
if (hasSquare) {
g.setColor(Color.RED);
g.fillRect(1,1,size-2,size-2);
}
g.dispose();
return bi;
}
public static void main(String[] args) {
SwingUtilities.invokeLater( new Runnable() {
public void run() {
Image selected = getImage(true);
Image unselected = getImage(false);
int row = 2;
int col = 5;
JPanel p = new JPanel(new GridLayout(row,col));
for (int ii=0; ii<row*col; ii++) {
p.add( getButton(selected, unselected) );
}
JOptionPane.showMessageDialog(null, p);
}
});
}
}
Note that a button will react to both mouse and keyboard input, whereas (by default) a label won't.