OBJECTIVE-C
Here what we are going to achieve is a reverse countdown timer with circular progress.
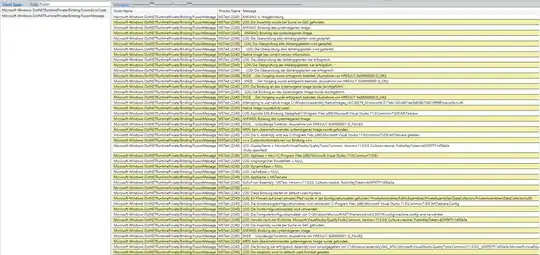
We need to follow some steps to achieve the above result in Objective-C.
A. Create XIB Files.
B. Design the layout
C. Configure the circular progress with this library. MBCircularProgressBarView
D. Populate XIB in the UIViewController.
==============================================================
A. Create XIB Files:
- Go to Project Navigation and by right click, you will get the options to create a new file ->
Cocoa Touch Class
-> write Class
Name: ProgressDelayView
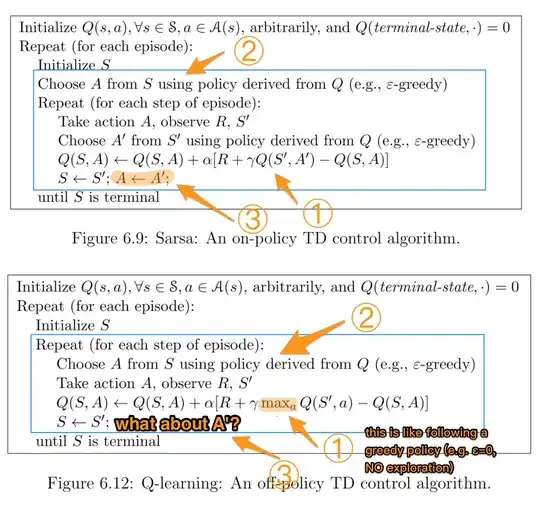
You will get the .h
& .m
file named ProgressDelayView
Now we will create a User Interface for the class files.
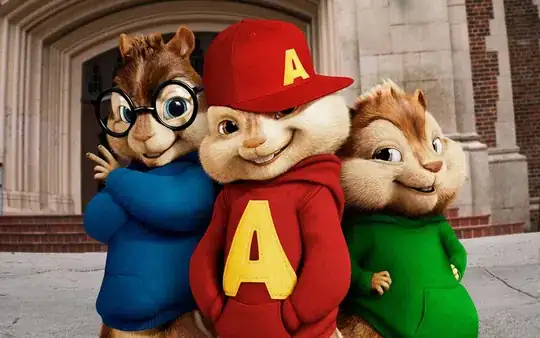
This time you will get the .xib
file named ProgressDelayView
Now, Assign the class name to the Interface file
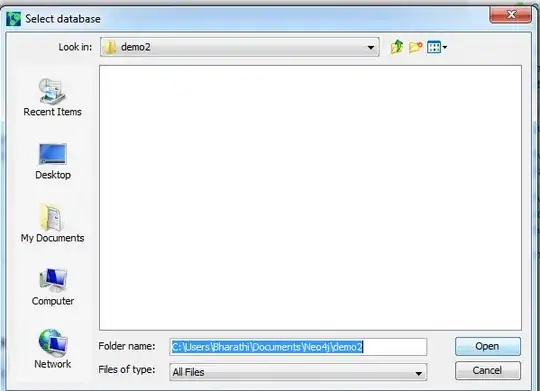
B. Design the layout
Now design the layout according to your requirement. I have designed based on mine.
C. Configure the circular progress with this library. MBCircularProgressBarView
Assign the attribute properties based on your app theme.
please check out the attributes in a screenshot.
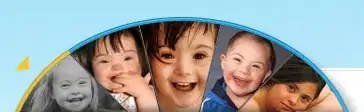
D. Populate XIB in the UIViewController.
Let's move to the code part
Open your viewcontroller.h
file
and paste this code.
#import <UIKit/UIKit.h>
@interface viewcontroller : UIViewController
@property (nonatomic) int remainingSeconds;
@end
open viewcontroller.m
and paste this
#import "viewcontroller.h"
#import "ProgressDelayView.h"
@interface viewcontroller(){
ProgressDelayView *TimerTicker;
NSTimer *timer;
}
@implementation viewcontroller
#pragma mark - Controller Lifecycle
- (void)viewDidLoad {
[super viewDidLoad];
TimerTicker = [[[NSBundle mainBundle] loadNibNamed:@"ProgressDelayView" owner:self options:nil] objectAtIndex:0];
}
-(void)viewDidAppear:(BOOL)animated {
[super viewDidAppear:animated];
[self populateTimeTicker];
}
-(void)populateTimeTicker {
TimerTicker.frame = CGRectMake(0, 0, self.view.frame.size.width, self.view.frame.size.height);
[self showWithAnimation:TimerTicker];
self.remainingSeconds = 180;
[self startTicker];
}
-(void)startTicker {
timer=[NSTimer scheduledTimerWithTimeInterval:1 target:self selector:@selector(timerTicker) userInfo:nil repeats:YES];
}
- (void)timerTicker {
self.remainingSeconds--;
if (self.remainingSeconds == -1) {
//dismiss alertview
[timer invalidate];
[TimerTicker removeFromSuperview];
return;
}
[UIView animateWithDuration:1.f animations:^{
TimerTicker.progress.value = self.remainingSeconds;
}];
}
-(void)showWithAnimation:(UIView *)customView{
[UIView animateWithDuration:0.5
delay:0.1
options: UIViewAnimationOptionCurveEaseIn
animations:^{
customView.frame = CGRectMake(0, 0, self.view.frame.size.width, self.view.frame.size.height);
}
completion:^(BOOL finished){
}];
[self.view addSubview:customView];
}
==============================================================
Check out these two files ProgressDelayView.h & ProgressDelayView.m
ProgressDelayView.h
#import <UIKit/UIKit.h>
#import "MBCircularProgressBarView.h"
NS_ASSUME_NONNULL_BEGIN
@interface ProgressDelayView : UIView
@property (weak, nonatomic) IBOutlet MBCircularProgressBarView *progress;
@end
NS_ASSUME_NONNULL_END
ProgressDelayView.m
#import "ProgressDelayView.h"
@implementation ProgressDelayView
@end
HURRAY hit the ⌘R