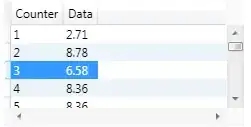
This is a simple code that get the input from the inputBox
to myText
. It should get you started on the right direction. Depending on what else you need to check or do, you can add more functions to it. Notice you might have to play around with the order of the line image = tk.PhotoImage(data=b64_data)
. Because if you put it right after b64_data = ...
. It will gives you error. (I am running MAC 10.6 with Python 3.2). And the picture only works with GIF at the moment. See reference at the bottom if you want to learn more.
import tkinter as tk
import urllib.request
import base64
# Download the image using urllib
URL = "http://www.contentmanagement365.com/Content/Exhibition6/Files/369a0147-0853-4bb0-85ff-c1beda37c3db/apple_logo_50x50.gif"
u = urllib.request.urlopen(URL)
raw_data = u.read()
u.close()
b64_data = base64.encodestring(raw_data)
# The string you want to returned is somewhere outside
myText = 'empty'
def getText():
global myText
# You can perform check on some condition if you want to
# If it is okay, then store the value, and exist
myText = inputBox.get()
print('User Entered:', myText)
root.destroy()
root = tk.Tk()
# Just a simple title
simpleTitle = tk.Label(root)
simpleTitle['text'] = 'Please enter your input here'
simpleTitle.pack()
# The image (but in the label widget)
image = tk.PhotoImage(data=b64_data)
imageLabel = tk.Label(image=image)
imageLabel.pack()
# The entry box widget
inputBox = tk.Entry(root)
inputBox.pack()
# The button widget
button = tk.Button(root, text='Submit', command=getText)
button.pack()
tk.mainloop()
Here is the reference if you want to know more about the Tkinter Entry Widget: http://effbot.org/tkinterbook/entry.htm
Reference on how to get the image: Stackoverflow Question