With kudos to @nwinkler's response, the main problem is matching the version number.
A common scenario is that if you are developing a set of projects that are version lock-steped with each other - for example, a project and a set of library projects that are not very loosely coupled, such that a library API might change in a version to be consumed by the relevant app project version, but may change in a way that is incompatible with a past or future version of the app project.
The correct way to set Maven dependencies in such a configuration (and it is also the recommended practice) is to have the app consume specific versions of the libraries - so, for example, if you rebuild an old version of the app, it will use the library version that it previously compiled with.
With the app project's POM library dependency set to a release version (lets say 1.0.0
), and while working on the next release with both the app and library projects set to a SNAPSHOT release (lets say 2.0.0-SNAPSHOT
), the m2e will not resolve the library version correctly, and will likely download an old version, so that trying to use Eclipse features like "Open Decleration" will target the download jar (sometimes without even a source attachment) which can be pretty annoying.
One way to work around that is to set the app POM dependency version to a range, so instead of depending on 1.0.0
, you'd depend on [1.0.0-)
. With an open range like that, m2e will happily find your workspace library project. But you'd want to set it back to the "correct" version before committing, building and publishing - and this can be very error prone.
My solution is to use build profiles and set a custom profile for m2e, like this:
- Set your dependency version with a property, to the version you want to publish against:
...
<properties>
<my.library.version>1.0.0</my.library.version>
</properties>
<dependencies>
<dependency>
<groupId>my.group</groupId>
<artifactId>my.library</artifactId>
<version>${my.library.version}</version>
</dependency>
</dependencies>
...
- Then add a profile section with an active by default profile that does nothing, and an Eclipse-specific profile that overrides the library version property with a range:
...
<profiles>
<profile>
<id>default</id>
<activation><activeByDefault></activeByDefault></activation>
</profile>
<profile>
<id>eclipse</id>
<properties>
<my.library.version>[1,)</my.library.version>
</properties>
</profile>
</profiles>
...
- Finally go to your project properties, and under "Maven" type "eclipse" into "Active Maven Profiles":
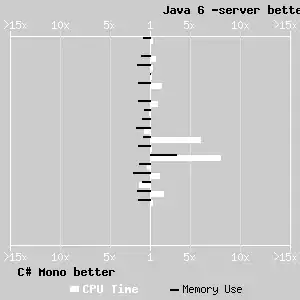
Eclipse m2e will then always see the version range and will resolve dependencies from the eclipse project (even if you have the library installed in the local Maven repo, as the Eclipse project will have a higher version number), but other builders will see the original, strict, version number.