// Wrap the list in a JScrollPane if 'size matters'.
JOptionPane.showMessageDialog(null, new JList(array));
E.G.
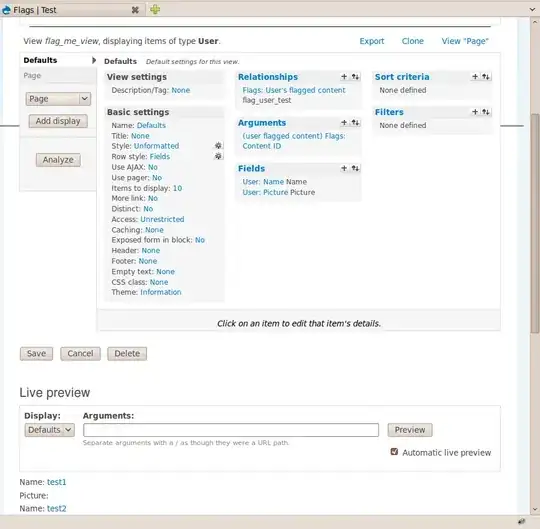
import javax.swing.*;
public class ArrayDisplay {
public static void main(String[] args) {
final String[] array = {
"JList",
"JTable for 2D array",
"HTML in JLabel",
"Delimited String in JLabel"
};
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
JOptionPane.showMessageDialog(null, new JList(array));
}
});
}
}
(In regard to array toString()
)
It gives garbage values.
Obscure, yes - garbage, no. AFAIU it is the reference to the array in memory.
..I have an array of type int
Note that a JList
array constructor requires objects, so it would need Integer
rather than int
. To convert from int[]
to Integer[]
do this:
import javax.swing.*;
public class ArrayDisplay {
public static void main(String[] args) {
int[] arrayPrimitive = {1,2,3};
final Integer[] array = new Integer[arrayPrimitive.length];
for (int ii=0; ii<arrayPrimitive.length; ii++) {
array[ii] = arrayPrimitive[ii];
}
SwingUtilities.invokeLater(new Runnable(){
@Override
public void run() {
JOptionPane.showMessageDialog( null, new JList(array) );
}
});
}
}