Well, let me try to explain with some images.
In Python, everything is an object. Those objects are referenced by variables. Some kinds of objects, such as lists and tuples, just store references to other objects.
That said, when you execute
myVar = ["jhhj", "hgc"]
myTuple = ([1,2,3], [4,5,6], myVar)
You get more or less this scenario:
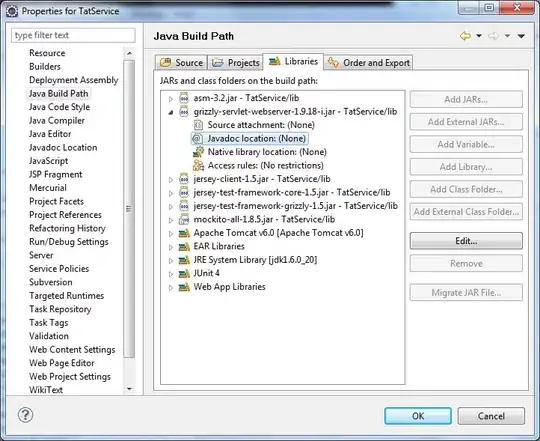
Each object is represented by a box/rectangle. We have two string objects, "jhhj"
and "hgc"
. Also, we have a list object, pointed by the variable myVar
; this list object points to both string objects. Also, we have a tuple object, referenced by myTuple
; this tuple object points two other lists and the list referenced by myVar
.
When you execute
myVar.append('lololol')
what does happen? Well, the list object (that is incidentally pointed by myVar
) starts to referenced one more value, the string object "lololol"
:
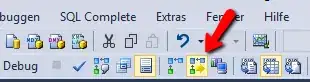
Note that myVar
still references the list object. What happened is that the list object changed. You can look at this list object both from myVar
or from the tuple, you will see the same object with the same change.
OTOH, when you execute
myVar = "lol"
myTuple = ([1,2,3], [4,5,6], myVar)
You get something like this:
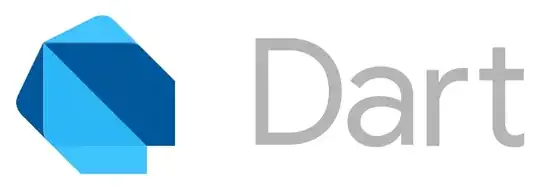
Now myVar
points to the string object "lol"
, as well the tuple references it at its third position. Now, if you execute
myVar = "lolol"
you are just making myVar
to point to another object. The tuple object still points to "lol"
as before:
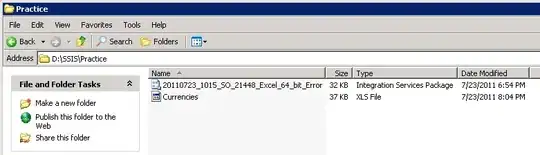
So, if you attribute a new value to a variable, it will just change the value pointed by this variable. The previous value referenced by the variable will still exist* and any other variable or object pointing to it will stay pointing to it. Just the attributed variable will change.
PS: Also, I answered a vaguely related question some time ago. You may find the answer helpful.
* Except if it is collected by the garbage collector, but this is another long history.