If you want to center the content horizontally and vertically, but don't know in prior how high your page will be, you have to you use JavaScript.
HTML:
<body>
<div id="content">...</div>
</body>
CSS:
#content {
max-width: 1000px;
margin: auto;
left: 1%;
right: 1%;
position: absolute;
}
JavaScript (using jQuery):
$(function() {
$(window).on('resize', function resize() {
$(window).off('resize', resize);
setTimeout(function () {
var content = $('#content');
var top = (window.innerHeight - content.height()) / 2;
content.css('top', Math.max(0, top) + 'px');
$(window).on('resize', resize);
}, 50);
}).resize();
});
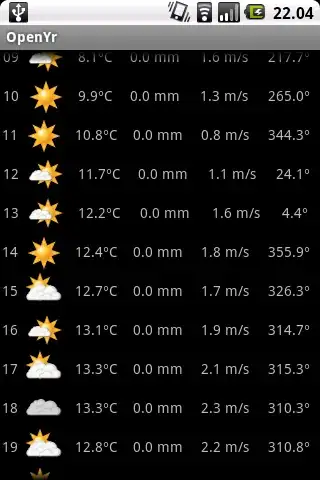
Demo: http://jsfiddle.net/nBzcb/