If you want to print color in the IDLE shell no answer using ASCI escape codes will help you as it does not implement this feature.
There is a hack specific to IDLE that lets you write to it's PyShell
object directly and specify text tags that IDLE has already defined such as "STRING"
which will appear as green by default.
import sys
try:
shell = sys.stdout.shell
except AttributeError:
raise RuntimeError("you must run this program in IDLE")
shell.write("Wanna go explore? ","KEYWORD")
shell.write("OPTIONS","STRING")
shell.write(" : ","KEYWORD")
shell.write("Yes","DEFINITION")
shell.write(" or ","KEYWORD")
shell.write("No","COMMENT")
answer = input()
When run in IDLE will result in this prompt:
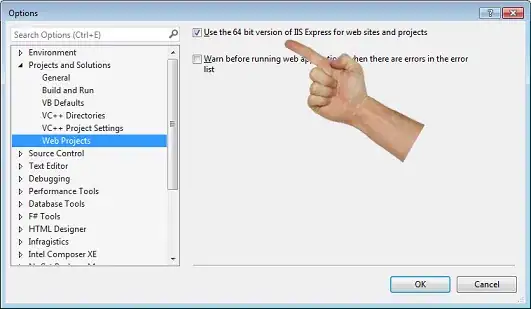
Here is a list of all valid tags for use:
print("here are all the valid tags:\n")
valid_tags = ('SYNC', 'stdin', 'BUILTIN', 'STRING', 'console', 'COMMENT', 'stdout',
'TODO','stderr', 'hit', 'DEFINITION', 'KEYWORD', 'ERROR', 'sel')
for tag in valid_tags:
shell.write(tag+"\n",tag)
Note that 'sel'
is special that it represents the text that is selected, so it will be un-selected once something else is clicked on. As well it can be used to start some text selected for copying.