It's pretty easy with a custom TextView
class. Check out the Canvas guide from the documentation for more info on this. Note the comments where you can change the color/width:
public class ObliqueStrikeTextView extends TextView
{
private int dividerColor;
private Paint paint;
public ObliqueStrikeTextView(Context context)
{
super(context);
init(context);
}
public ObliqueStrikeTextView(Context context, AttributeSet attrs)
{
super(context, attrs);
init(context);
}
public ObliqueStrikeTextView(Context context, AttributeSet attrs, int defStyle)
{
super(context, attrs, defStyle);
init(context);
}
private void init(Context context)
{
Resources resources = context.getResources();
//replace with your color
dividerColor = resources.getColor(R.color.black);
paint = new Paint();
paint.setColor(dividerColor);
//replace with your desired width
paint.setStrokeWidth(resources.getDimension(R.dimen.vertical_divider_width));
}
@Override
protected void onDraw(Canvas canvas)
{
super.onDraw(canvas);
canvas.drawLine(0, getHeight(), getWidth(), 0, paint);
}
}
You can use it in a layout file by fully qualifying the view with your package, like this:
<your.package.name.ObliqueStrikeTextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="1234567890"
android:textSize="20sp"/>
Here's the end result:
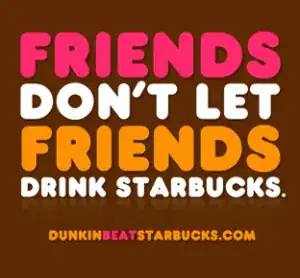