Typically when you are hosting your website (I'm assuming ASP .Net MVC here) on IIS your requests going to server side will mostly fall into only two categories of http verbs namely GET
or POST
. Have a look at below mentioned ASP .NET MVC code. At the controller level it is possible decorate our actions using any of the http verbs though:
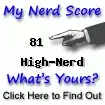
Now let's first understand if we are using plain vanilla ASP .NET MVC framework (without any java script) then why every request falls into only two categories namely GET
and POST
.
Let's say I've a view (aka a web page) in my website which is responsible for registering new users visiting my website. You will see controller code as below:
namespace MvcTestApp.Controllers
{
// GET: /Account/Register
[HttpGet]
public ActionResult Register()
{
return View();
}
//
// POST: /Account/Register
[HttpPost]
public ActionResult Register(RegisterModel model)
{
if (ModelState.IsValid)
{
// Attempt to register the user
try
{
WebSecurity.CreateUserAndAccount(model.UserName, model.Password);
WebSecurity.Login(model.UserName, model.Password);
return RedirectToAction("Index", "Home");
}
catch (MembershipCreateUserException e)
{
ModelState.AddModelError("", ErrorCodeToString(e.StatusCode));
}
}
// If we got this far, something failed, redisplay form
return View(model);
}
}
}
You type the http://localhost:9896/Account/Register/
url in your browser's address bar for loading the register view/web-page in your browser. That's like first request to the web page so your browser automatically defaults it to HttpGet
verb as you are getting the web page for the first time. So in this case below method annotated with [HttpGet]
verb gets called:
[HttpGet]
public ActionResult Register()
{
return View();
}
Now on the page I've a button which I click to initiate the registration process once I've provided all the details related to registration. If you look at the source of the web page then you see below html code for the button:
<input type="submit" value="Register" />
Whenever you click on an HTML control of type submit
its simple job is to post/submit the updated contents of the current page to the server. Any of the html controls including input
html control which make server side request are capable of making only HttpPost
verb requests through browser. Since it is an httpPost
verb request to the server for exactly the same URL the below mentioned method which is decorated with HttpPost
get called. ASP.Net run-time obtains this information from the client-request that it is actually an http POST request and hence decides to invoke the below method on the controller having [HttpPost]
annotation:
[HttpPost]
[AllowAnonymous]
[ValidateAntiForgeryToken]
public ActionResult Register(RegisterModel model)
{
if (ModelState.IsValid)
{
.....
.....
}
Thus as long as you are not using any javascript on your view/web page you ultimately make either http get (first-time) request or http post (using html submit controls) request.
Out of CRUD operations, Create and Update/Modify operations are handled by the same method annotated with [HttpPost]
.
HTTP put verb is used to create/replace a resource when two below conditions are satisfied:
- The endpoint must be idempotent: so safe to redo the request over and over again.
- The URI must be the address to the resource being updated.
You can read more about put vs post here.
So if you business scenario on server side will be able to manage the constraints related to HTTP put verb then ASP.NET MVC has a provision for that. You can create an action inside the controller and decorate it with [HttpPut]
and call it using using http put verb from browser.
Well, even if in some scenario where you happen to satisfy the criteria laid down by put verb then you will not be able to call it using HTTP submit button. You will have to resort to java script XMLHttpRequest (i.e. AJAX calls) to make put verb calls as shown below:
$.ajax({
url: '/Account/Register',
type: 'PUT',
success: function(result) {
// Do something with the result
}
});
Also, around the usage of http delete verb I've see people explicitly having an action defined on the MVC controller e.g. void DeleteUser(int userId)
which is called using http POST verb.
So in summary, we can see that for http PUT, POST and DELETE verbs we just have actions/methods on server side which are called using http POST verb. I've not come across a scenario in usual business applications where I ever got thinking to decorate my MVC actions with [HttpPut]
or [HttpDelete]
.
Hope this helps you get some clarification.