The reasoning behind the volatile
semantic is rooted in the Java Memory Model, which is specified in terms of actions:
- reads and writes to variables
- locks and unlocks of monitors
- starting and joining with threads
The Java Memory Model defines a partial ordering called happens-before for the actions which can occur in a Java program. Normally there is no guarantee, that threads can see the results of each other actions.
Let's say you have two actions A and B. In order to guarantee, that a thread executing action B can see the results of action A, there must be a happens-before relationship between A and B. If not, the JVM is free to reorder them as it likes.
A program which is not correctly synchronized might have data races. A data race occurs, when a variable is read by > 1 threads and written by >= 1 thread(s), but the read and write actions are not ordered through the happens-before ordering.
Hence, a correctly synchronized program has no data races, and all actions within the program happen in a fixed order.
So actions are generally only partially ordered, but there is also a total order between:
- lock acquisition and release
- reads and writes to volatile variables
These actions are totally ordered.
This makes it sensible to describe happens-before in terms of "subsequent" lock acquisitions and reads of volatile variables.
Regarding your questions:
- With the happen-before relationship you have an alternative definition of
volatile
- Reversing the order would not make sense to the definition above, especially since there is a total order involved.
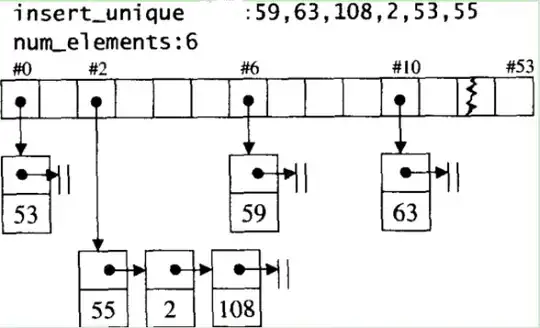
This illustrates the happens-before relation when two threads synchronize using a common lock. All the actions within thread A are ordered by the program order rule, as are the actions within thread B. Because A releases lock M and B subsequently acquires M, all the actions in A before releasing the lock are therefore ordered before the actions in B after acquiring the lock. When two threads synchronize on different locks, we can't say anything about the ordering of actions between themthere is no happens-before relation between the actions in the two threads.
Source: Java Concurrency in Practice