No, you don't have to do that, and you can call your function from anywhere.
Try this:
Put this code in Module1:
Sub TestSheetExists()
If SheetExists("Sheet1") Then
MsgBox "I exist!"
End If
End Sub
And this in Module2:
Function SheetExists(shtName As String, Optional wb As Workbook) As Boolean
Dim sht As Worksheet
If wb Is Nothing Then Set wb = ThisWorkbook
On Error Resume Next
Set sht = wb.Sheets(shtName)
On Error GoTo 0
SheetExists = Not sht Is Nothing
End Function
Obviously you can use whatever names for your modules you want.
EDIT: I see that calling from different modules still isn't working for you. Follow these steps exactly to set up a test workbook that should help you understand the problem.
- Create a new Excel workbook
- Open the VBA Editor (Alt-F11)
- Right-click on the project and select insert module. Repeat this 4x to get 4 modules.
- Press F4 to open the properties window, if it isn't already open
- Change your module names to the following: CallMe, CallMeAgain, CallMeBack, Validation
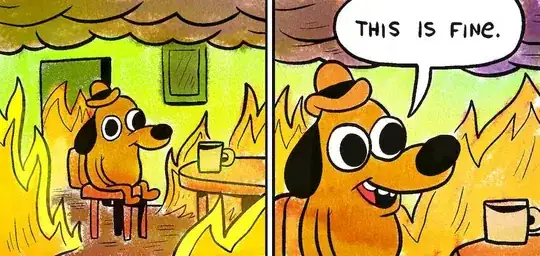
In the Validation module, paste the following function:
Function SheetExists(shtName As String, Optional wb As Workbook) As Boolean
Dim sht As Worksheet
If wb Is Nothing Then Set wb = ThisWorkbook
On Error Resume Next
Set sht = wb.Sheets(shtName)
On Error GoTo 0
SheetExists = Not sht Is Nothing
End Function
Paste this sub into CallMe:
Sub TestSheetExistsFromCallMe()
If SheetExists("Sheet1") Then
MsgBox "I exist, and I was called from CallMe!"
End If
End Sub
Paste this into CallMeBack:
Sub TestSheetExistsFromCallMeBack()
If SheetExists("Sheet1") Then
MsgBox "I exist, and I was called from CallMeBack!"
End If
End Sub
Paste this into CallMeAgain:
Sub TestSheetExistsFromCallMeAgain()
If SheetExists("Sheet1") Then
MsgBox "I exist, and I was called from CallMeAgain!"
End If
End Sub
Press F5 to run the code from within CallMe. You should see the following messagebox:
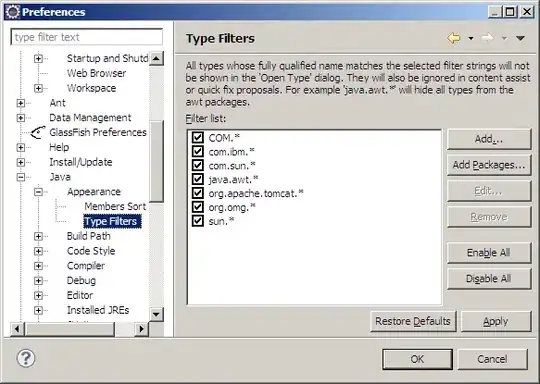
Run the code from any of the 3 "Call" modules and you should see the corresponding messagebox.
I got the SheetExists function from Tim Williams (https://stackoverflow.com/a/6688482/138938) and use it all the time.