The method everyone will recommend, which sadly won't work in all cases..
You could traverse up the node-tree using the elements parent-property, checking if there is any element that has an explicit background-color.
The problem with this approach is that elements set to reside outside of their parent (using for example position:relative with the appropriate sibling values) can't be identified.
Any portable and 100% working solution to the problem?
The only portable way I can think of that will always yield the correct result is to find the (x,y) of where your element is in the browser.
Then iterate over all elements to see if any elements (x,y) results in that it's behind the needle-element and then check whether this element has a background-color property or not.
This is guaranteed to work, but will have a very hard impact on performance. If you are sure of the fact that an element isn't going to float itself outside of the bounds of it's true parent use the first method described.
If you'd like this method to work for elements which have an image as background-property you could load the said image onto a canvas and read the value of the pixel you'd like, though that will make the already performance sucking operation even more sucking, but it will work.
Also;
- what if an element is floating above more than one element?
- which color should be returned in a function implementing what you are describing?
- The color shining through in the center of your needle-element, or maybe in the top left corner?
Element floating over 4 different elements, which is the elements background color?
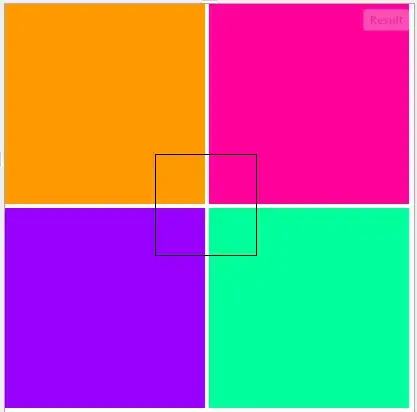
nobody knows..