Create some kind of container class (that is some kind of collection) that stores your key-value pairs, and bind them to a DataGrid
at runtime.
Quick'n'dirty example:
Class Container
Inherits BindingList(Of Value)
Class Value
Public Property Key As String
Public Property Value As Object
End Class
Public Sub New()
Add(New Value With {.Key = "Name"})
Add(New Value With {.Key = "Age"})
Add(New Value With {.Key = "Place"})
End Sub
Default Public Overloads Property Item(ByVal key As String) As Object
Get
Return Me.FirstOrDefault(Function(v) v.Key = key)
End Get
Set(ByVal value As Object)
Dim v = Me.FirstOrDefault(Function(e) e.Key = key)
If v Is Nothing Then
Add(New Value With {.Key = key, .Value = value})
Else
v.Value = value
End If
End Set
End Property
End Class
In your Form
:
Private Sub Form1_Load(sender As System.Object, e As System.EventArgs) Handles MyBase.Load
Dim grd = New DataGrid With {.Dock = DockStyle.Fill}
Controls.Add(grd)
Dim container = New Container()
grd.DataSource = container
container("Age") = 12
container("Place") = "Somewhere"
End Sub
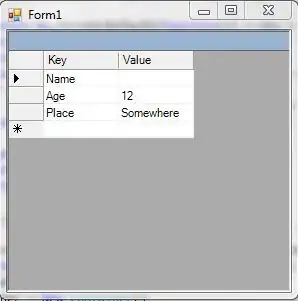
You then have to adjust the apperance of your DataGrid of course, it's up to you.
This way, the grid is bound to the container
object, and you can read/change values easily.