It's a little difficult to understand what it is you're trying to achieve from your post, but if you're interested in knowing when the editor content is changed you could try
final JComboBox combo = new JComboBox();
combo.setEditable(true);
((JTextComponent) combo.getEditor().getEditorComponent()).getDocument().addDocumentListener(new DocumentListener() {
protected void updatePopup() {
if (combo.isDisplayable()) {
if (!combo.isPopupVisible()) {
combo.showPopup();
}
}
}
@Override
public void insertUpdate(DocumentEvent e) {
updatePopup();
}
@Override
public void removeUpdate(DocumentEvent e) {
updatePopup();
}
@Override
public void changedUpdate(DocumentEvent e) {
updatePopup();
}
});
Normally, I'd create a "DocumentHandler" as a concrete class and use that instead, but the example demonstrates the basic idea
UPDATED with a UI example
public class TestComboBox extends JFrame {
public TestComboBox() {
setTitle("Test");
setSize(200, 200);
setLayout(new GridBagLayout());
setDefaultCloseOperation(EXIT_ON_CLOSE);
setLocationRelativeTo(null);
final JComboBox combo = new JComboBox();
/**** You have to do this first
**** Doing this invalidates any previous listeners
****/
combo.setUI(ColorArrowUI.createUI(combo));
combo.setEditable(true);
((JTextComponent) combo.getEditor().getEditorComponent()).getDocument().addDocumentListener(new DocumentListener() {
protected void updatePopup() {
if (combo.isDisplayable()) {
if (!combo.isPopupVisible()) {
combo.showPopup();
}
}
}
@Override
public void insertUpdate(DocumentEvent e) {
updatePopup();
}
@Override
public void removeUpdate(DocumentEvent e) {
updatePopup();
}
@Override
public void changedUpdate(DocumentEvent e) {
updatePopup();
}
});
combo.setModel(createComboBoxModel());
add(combo);
setVisible(true);
}
protected ComboBoxModel createComboBoxModel() {
DefaultComboBoxModel model = new DefaultComboBoxModel();
File file = new File("../TestWords/Words.txt");
BufferedReader reader = null;
try {
reader = new BufferedReader(new FileReader(file));
String text = null;
while ((text = reader.readLine()) != null) {
model.addElement(text);
}
} catch (Exception e) {
} finally {
try {
reader.close();
} catch (Exception e) {
}
}
return model;
}
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
} catch (InstantiationException ex) {
} catch (IllegalAccessException ex) {
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
}
new TestComboBox();
}
public static class ColorArrowUI extends BasicComboBoxUI {
public static ComboBoxUI createUI(JComponent c) {
return new ColorArrowUI();
}
@Override
protected JButton createArrowButton() {
return new BasicArrowButton(
BasicArrowButton.SOUTH,
Color.cyan, Color.magenta,
Color.yellow, Color.blue);
}
}
}
Set using the UI
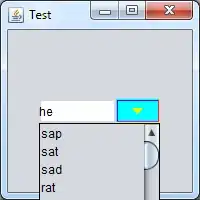
Set using the painter
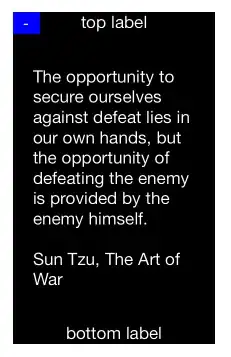
Updated
This is the code that kleopatra showed you
Painter painter = new Painter<JComponent>() {
@Override
public void paint(Graphics2D g, JComponent object, int width, int height) {
g.setColor(Color.WHITE);
g.fillRect(0, 0, width, height);
}
};
JButton org = null;
for (int i = 0; i < combo.getComponentCount(); i++) {
if (combo.getComponent(i) instanceof JButton) {
org = (JButton) combo.getComponent(i);
UIDefaults buttonDefaults = new UIDefaults();
buttonDefaults.put("ComboBox:\"ComboBox.arrowButton\"[Enabled].foregroundPainter", painter);
org.putClientProperty("Nimbus.Overrides.InheritDefaults", false);
org.putClientProperty("Nimbus.Overrides", buttonDefaults);
break;
}
}