I realize I am a little late to this party, but why not just add the CustomDelegate class to your VC in Interface Builder and set the delegate for the UITextField
to that? No extra code in the VC is required, no UITextField
subclassing is needed either. Project link below that does this to custom format a phone number.
Link to example project This link will go away when I decide it will go away. It could live on here forever (or as long as Dropbox is in business).
Added code example of the delegate class (although if someone had any amount of experience in iOS development, this wouldn't be needed.) That screenshot explains just about all of the answer. Example project link added as a convenience.
Code from the Delegate class:
Header File:
#import <Foundation/Foundation.h>
@interface PhoneNumberFormatterDelegate : NSObject <UITextFieldDelegate>
@end
Implementation File:
#import "PhoneNumberFormatterDelegate.h"
@implementation PhoneNumberFormatterDelegate
#pragma mark - My Methods
-(void)textFieldDidChange:(UITextField *)textField {
if ([textField.text length] > 11) {
[textField resignFirstResponder];
}
}
#pragma mark - UITextField Delegate Methods
- (BOOL)textField:(UITextField *)textField shouldChangeCharactersInRange:(NSRange)range replacementString:(NSString *)string {
// If user hit the Done button, resign first responder
if([string isEqualToString:@"\n"]){
[textField resignFirstResponder];
return NO;
}
// All digits entered
if (range.location == 12) {
[textField resignFirstResponder];
return NO;
}
// Reject appending non-digit characters
if (range.length == 0 &&
![[NSCharacterSet decimalDigitCharacterSet] characterIsMember:[string characterAtIndex:0]]) {
return NO;
}
// Auto-add hyphen before appending 4rd or 7th digit
if (range.length == 0 &&
(range.location == 3 || range.location == 7)) {
textField.text = [NSString stringWithFormat:@"%@-%@", textField.text, string];
return NO;
}
// Delete hyphen when deleting its trailing digit
if (range.length == 1 &&
(range.location == 4 || range.location == 8)) {
range.location--;
range.length = 2;
textField.text = [textField.text stringByReplacingCharactersInRange:range withString:@""];
return NO;
}
return YES;
}
- (void)textFieldDidBeginEditing:(UITextField *)textField {
[textField addTarget:self action:@selector(textFieldDidChange:) forControlEvents:UIControlEventEditingChanged];
}
- (void)textFieldDidEndEditing:(UITextField *)textField {
[textField removeTarget:self action:@selector(textFieldDidChange:) forControlEvents:UIControlEventEditingChanged];
}
@end
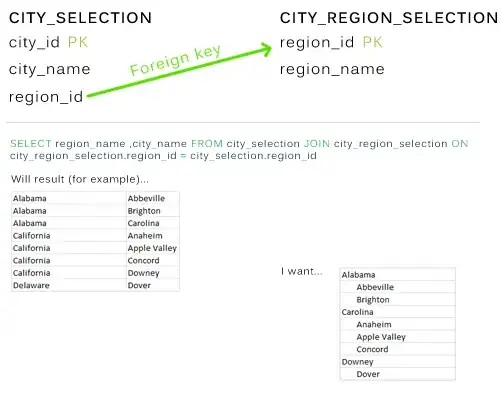