tl;dr
LocalDate.parse( "2012-07-27" )
.toString()
2012-07-27
Details
The modern way is with the java.time classes.
The LocalDate
class represents a date-only value without time-of-day and without time zone.
Standard format
Your input string format happens to comply with the ISO 8601 standard. The java.time classes use ISO 8601 formats by default when parsing/generating strings that represent date-time values. So no need to specify a formatting pattern at all.
LocalDate ld = LocalDate.parse( "2012-07-27" );
Do not conflate date-time objects with Strings representing their value. A date-time class/object can parse a string and generate a string, but the String
is always a separate and distinct object.
String output = ld.toString();
2012-07-27
Custom format
If your input is non-standard, define a DateTimeFormatter
to match.
String input = "20-12-2005" ;
DateTimeFormatter f = DateTimeFormatter.ofPattern( "dd-MM-uuuu" ) ;
LocalDate ld = LocalDate.parse( input , f ) ;
ld.toString(): 2012-07-27
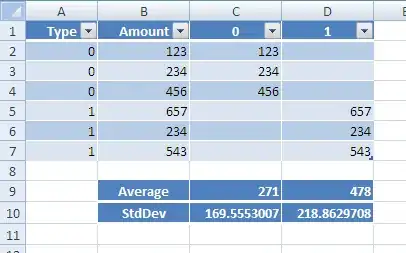
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
Where to obtain the java.time classes?
- Java SE 8 and SE 9 and later
- Built-in.
- Part of the standard Java API with a bundled implementation.
- Java 9 adds some minor features and fixes.
- Java SE 6 and SE 7
- Much of the java.time functionality is back-ported to Java 6 & 7 in ThreeTen-Backport.
- Android
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.