I am not quite sure if I understood what you mean. My initial understanding was similar to @sergio - how to implement a gesture recognizer. But it seems you are looking for "how to design" your MVC (based on your comment to @sergio : "...My issue is actually getting the array and everything passed over...".
If so, here is a suggestion that might be helpful:
If you refactor your model, all entries, into its own class (subclass of NSObject
) and declare some convenient properties, so that when, let's say, EntriesViewController
pushes WebViewController
into the navigation stack, instead of passing the entry itself, you can pass a pointer to the model. WebViewController
can make its own inquiries to model.
Something like this diagram:
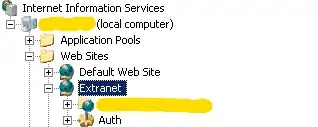
Hope this helps!
Edit:
Here is your model interface
#import <Foundation/Foundation.h>
@interface SARSSEntryModel : NSObject
@property (nonatomic, strong) NSMutableArray *allEntries;
@property (nonatomic) NSUInteger currentEntryIndex;
@end
Here is model implementation
#import "SARSSEntryModel.h"
@implementation SARSSEntryModel
@synthesize allEntries = _allEntries;
@synthesize currentEntryIndex = _currentEntryIndex;
// instantiate on-demand
- (NSMutableArray *)allEntries {
if (!_allEntries) {
_allEntries = [[NSMutableArray alloc] initWithObjects:@"http://www.google.com", @"http://www.yahoo.com", @"http://www.bing.com", nil];
}
return _allEntries;
}
Here is EntriesViewController interface
#import <UIKit/UIKit.h>
#import "SARSSEntryModel.h"
#import "SAWebViewController.h"
@interface SAEntriesViewController : UITableViewController
@property (nonatomic, strong) SARSSEntryModel *model; // this is your model
@end
Here is EntriesViewController implementation
#import "SAEntriesViewController.h"
@implementation SAEntriesViewController
@synthesize model = _model;
- (void)viewDidLoad {
self.model = [[SARSSEntryModel alloc] init];
[super viewDidLoad];
}
- (void)viewDidUnload {
[self setModel:nil];
[super viewDidUnload];
}
#pragma mark - Table view data source
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
return self.model.allEntries.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
static NSString *CellIdentifier = @"cell identifier";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier];
// Configure the cell...
cell.textLabel.text = [self.model.allEntries objectAtIndex:indexPath.row];
return cell;
}
#pragma mark - Table view delegate
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath {
self.model.currentEntryIndex = indexPath.row;
SAWebViewController *wvc = [self.storyboard instantiateViewControllerWithIdentifier:@"WebViewControllerIdentifier"];
wvc.model = self.model;
[self.navigationController pushViewController:wvc animated:YES];
}
Here is WebViewController interface
#import <UIKit/UIKit.h>
#import "SARSSEntryModel.h"
@interface SAWebViewController : UIViewController
@property (nonatomic, strong) SARSSEntryModel *model;
@property (weak, nonatomic) IBOutlet UIWebView *webView;
@end
Here is WebViewController implementation
#import "SAWebViewController.h"
@implementation SAWebViewController
@synthesize webView = _webView;
@synthesize model = _model;
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view.
// for testing. to prevent conflict with one touch swipe
UISwipeGestureRecognizer *swipe = [[UISwipeGestureRecognizer alloc] initWithTarget:self action:@selector(navigate:)];
[self.webView addGestureRecognizer:swipe];
swipe = nil;
}
- (void)viewDidUnload {
[self setWebView:nil];
[self setModel:nil];
[super viewDidUnload];
// Release any retained subviews of the main view.
}
- (void)viewDidAppear:(BOOL)animated {
[self loadURL];
[super viewDidAppear:animated];
}
- (void)loadURL {
NSString *urlString = [self.model.allEntries objectAtIndex:self.model.currentEntryIndex];
NSURL *url = [NSURL URLWithString:urlString];
NSURLRequest *request = [[NSURLRequest alloc] initWithURL:url];
[self.webView loadRequest:request];
}
- (void)navigate:(id)sender {
UISwipeGestureRecognizer *swipe = (UISwipeGestureRecognizer *)sender;
if (swipe.direction == UISwipeGestureRecognizerDirectionLeft) {
if (self.model.currentEntryIndex < self.model.allEntries.count) {
self.model.currentEntryIndex++;
}
else {
self.model.currentEntryIndex = 0;
}
}
else if (swipe.direction == UISwipeGestureRecognizerDirectionRight) {
if (self.model.currentEntryIndex > 0) {
self.model.currentEntryIndex--;
}
else {
self.model.currentEntryIndex = self.model.allEntries.count - 1;
}
}
[self loadURL];
}
@end