XxxTableModel
stores String.class
for JButton
as Renderer
/ Editor
for JTable
and for your code (is based on) too
EDIT
DefaultTableModel
import java.awt.event.*;
import java.util.*;
import javax.swing.*;
import javax.swing.table.*;
public class TableButton3 extends JFrame {
private static final long serialVersionUID = 1L;
private JTable table;
public TableButton3() {
String[] columnNames = {"Date", "String", "Decimal", "Remove"};
Object[][] data = {
{new Date(), "A", new Double(5.1), "Remove"}, {new Date(), "B", new Double(6.2), "Remove"},
{new Date(), "C", new Double(7.3), "Remove"}, {new Date(), "D", new Double(8.4), "Remove"},
{new Date(), "A", new Double(5.1), "Remove"}, {new Date(), "B", new Double(6.2), "Remove"},};
DefaultTableModel model = new DefaultTableModel(data, columnNames){
private static final long serialVersionUID = 1L;
@Override// Returning the Class of each column will allow different renderers to be used based on Class
public Class getColumnClass(int column) {
switch (column) {
case 0:
return Date.class;
case 2:
return Double.class;
default:
return String.class;
}
//return getValueAt(0, column).getClass();
}
};
table = new JTable(model) ;
table.setPreferredScrollableViewportSize(table.getPreferredSize());
JScrollPane scrollPane = new JScrollPane(table);
getContentPane().add(scrollPane);
ButtonColumn buttonColumn = new ButtonColumn(table, delete, 3);
buttonColumn.setMnemonic(KeyEvent.VK_D);
}
public static void main(String[] args) {
TableButton3 frame = new TableButton3();
frame.setDefaultCloseOperation(EXIT_ON_CLOSE);
frame.pack();
frame.setVisible(true);
}
//
private Action delete = new AbstractAction() {
private static final long serialVersionUID = 1L;
public void actionPerformed(ActionEvent e) {
JTable table = (JTable) e.getSource();
int modelRow = Integer.valueOf(e.getActionCommand());
((DefaultTableModel) table.getModel()).removeRow(modelRow);
table.clearSelection();
}
};
}
AbstractTableModel
import java.awt.event.*;
import java.util.*;
import javax.swing.*;
import javax.swing.table.*;
public class TableButton3 extends JFrame {
private static final long serialVersionUID = 1L;
private JTable table;
private MyTableModel myModel = new MyTableModel();
public TableButton3() {
String[] columnNames = {"Date", "String", "Decimal", "Remove"};
Object[][] data = {
{new Date(), "A", new Double(5.1), "Remove"}, {new Date(), "B", new Double(6.2), "Remove"},
{new Date(), "C", new Double(7.3), "Remove"}, {new Date(), "D", new Double(8.4), "Remove"},
{new Date(), "A", new Double(5.1), "Remove"}, {new Date(), "B", new Double(6.2), "Remove"},};
DefaultTableModel model = new DefaultTableModel(data, columnNames){
private static final long serialVersionUID = 1L;
@Override// Returning the Class of each column will allow different renderers to be used based on Class
public Class getColumnClass(int column) {
switch (column) {
case 0:
return Date.class;
case 2:
return Double.class;
default:
return String.class;
}
//return getValueAt(0, column).getClass();
}
};
table = new JTable(model);
table = new JTable(myModel);
addTableDatas();
table.setPreferredScrollableViewportSize(table.getPreferredSize());
JScrollPane scrollPane = new JScrollPane(table);
add(scrollPane);
ButtonColumn buttonColumn = new ButtonColumn(table, delete, 3);
buttonColumn.setMnemonic(KeyEvent.VK_D);
}
public static void main(String[] args) {
TableButton3 frame = new TableButton3();
frame.setDefaultCloseOperation(EXIT_ON_CLOSE);
frame.pack();
frame.setVisible(true);
}
private void addTableDatas() {
Vector<String> columnNameS = new Vector<String>();
columnNameS.add("Date");
columnNameS.add("String");
columnNameS.add("Decimal");
columnNameS.add("Remove");
myModel.setColumnNames(columnNameS);
Vector<Object> row1 = new Vector<Object>();
row1.add(new Date());
row1.add("A");
row1.add(new Double(5.1));
row1.add("Remove");
myModel.addRow(row1);
row1 = new Vector<Object>();
row1.add(new Date());
row1.add("B");
row1.add(new Double(6.2));
row1.add("Remove");
myModel.addRow(row1);
row1 = new Vector<Object>();
row1.add(new Date());
row1.add("B");
row1.add(new Double(8.4));
row1.add("Remove");
myModel.addRow(row1);
row1 = new Vector<Object>();
row1.add(new Date());
row1.add("B");
row1.add(new Double(5.1));
row1.add("Remove");
myModel.addRow(row1);
row1 = new Vector<Object>();
row1.add(new Date());
row1.add("B");
row1.add(new Double(6.2));
row1.add("Remove");
myModel.addRow(row1);
}
private class MyTableModel extends AbstractTableModel {
private static final long serialVersionUID = 1L;
private Vector<Vector<Object>> data;
private Vector<String> colNames;
private boolean[] _columnsVisible = {true, true, true, true};
public MyTableModel() {
this.colNames = new Vector<String>();
this.data = new Vector<Vector<Object>>();
}
public MyTableModel(Vector<String> colnames) {
this.colNames = colnames;
this.data = new Vector<Vector<Object>>();
}
public void resetTable() {
this.colNames.removeAllElements();
this.data.removeAllElements();
}
public void setColumnNames(Vector<String> colNames) {
this.colNames = colNames;
this.fireTableStructureChanged();
}
public void addRow(Vector<Object> data) {
this.data.add(data);
this.fireTableRowsInserted(data.size() - 1, data.size() - 1);
}
public void removeRowAt(int row) {
this.data.removeElementAt(row);
this.fireTableRowsDeleted(row - 1, data.size() - 1);
}
@Override
public int getColumnCount() {
return this.colNames.size();
}
@Override
public Class<?> getColumnClass(int colNum) {
switch (colNum) {
case 0:
return Date.class;
case 2:
return Double.class;
default:
return String.class;
}
}
@Override
public boolean isCellEditable(int row, int colNum) {
switch (colNum) {
default:
return true;
}
}
@Override
public String getColumnName(int colNum) {
return this.colNames.get(colNum);
}
@Override
public int getRowCount() {
return this.data.size();
}
@Override
public Object getValueAt(int row, int col) {
Vector<Object> value = this.data.get(row);
return value.get(col);
}
@Override
public void setValueAt(Object newVal, int row, int col) {
Vector<Object> aRow = data.elementAt(row);
aRow.remove(col);
aRow.insertElementAt(newVal, col);
fireTableCellUpdated(row, col);
}
public void setColumnVisible(int index, boolean visible) {
this._columnsVisible[index] = visible;
this.fireTableStructureChanged();
}
}
//
private Action delete = new AbstractAction() {
private static final long serialVersionUID = 1L;
public void actionPerformed(ActionEvent e) {
JTable table = (JTable) e.getSource();
int modelRow = Integer.valueOf(e.getActionCommand());
((DefaultTableModel) table.getModel()).removeRow(modelRow);
table.clearSelection();
}
};
}
both returns the same GUI, with the same ...
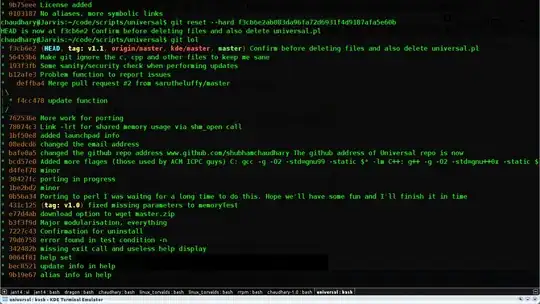