Python always uses pass by reference values. There is no exception. Any variable assignment means assigning the reference value. No exception. Any variable is the name bound to the reference value. Always.
You can think about reference as about the address of the target object that is automatically dereferenced when used. This way it seems you work directly with the target object. But there always is a reference in between, one step more to jump to the target.
Updated -- here is a wanted example that proves passing by reference:
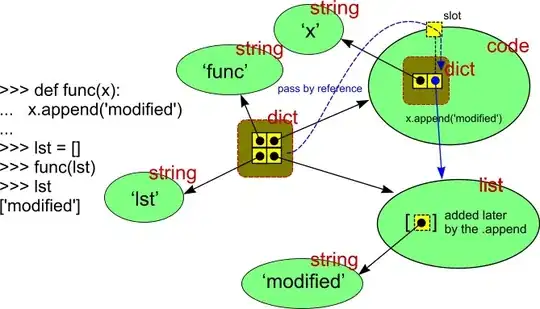
If the argument were passed by value, the outer lst
could not be modified. The green are the target objects (the black is the value stored inside, the red is the object type), the yellow is the memory with the reference value inside -- drawn as the arrow. The blue solid arrow is the reference value that was passed to the function (via the dashed blue arrow path). The uggly dark yellow is the internal dictionary. (It actually could be drawn also as a green elipse. The colour and the shape only says it is internal.)
Updated -- related to fgb's comment on passing by reference example swap(a, b)
and the delnan's comment on imposibility to write the swap
.
In compiled languages, variable is a memory space that is capable to capture the value of the type. In Python, variable is a name (captured internally as a string) bound to the reference variable that holds the reference value to the target object. The name of the variable is the key in the internal dictionary, the value part of that dictionary item stores the reference value to the target.
The purpose of the swap
in other languages is to swap the content of the passed variables, i.e. swap the content of the memory spaces. This can be done also for Python, but only for variables that can be modified--meaning the content of their memory space can be modified. This holds only for modifiable container types. A simple variable in that sense is always constant, even though its name can be reused for another purpose.
If the function should create some new object, the only way to get it outside is or via a container type argument, or via the Python return
command. However, the Python return
syntactically look as if it was able to pass outside more than one argument. Actually, the multiple values passed outside form a tuple, but the tuple can be syntactically assigned to more outer Python variables.
Update related to the simulation of variables as they are perceived in other languages. The memory space is simulated by a single-element lists -- i.e. one more level of indirection. Then the swap(a, b)
can be written as in other languages. The only strange thing is that we have to use the element of the list as the reference to the value of the simulated variable. The reason for neccessity to simulate the other-languate variables this way is that only containers (a subset of them) are the only objects in Python that can be modified:
>>> def swap(a, b):
... x = a[0]
... a[0] = b[0]
... b[0] = x
...
>>> var1 = ['content1']
>>> var2 = ['content2']
>>> var1
['content1']
>>> var2
['content2']
>>> id(var1)
35956296L
>>> id(var2)
35957064L
>>> swap(var1, var2)
>>> var1
['content2']
>>> var2
['content1']
>>> id(var1)
35956296L
>>> id(var2)
35957064L
Notice that the now the var1
and var2
simulate the look of "normal" variables in classic languages. The swap
changes their content, but the addresses remain the same.
For modifiable object--as the lists are for example--you can write exactly the same swap(a, b)
as in other languages:
>>> def swap(a, b):
... x = a[:]
... a[:] = b[:]
... b[:] = x[:]
...
>>> lst1 = ['a1', 'b1', 'c1']
>>> lst2 = ['a2', 'b2', 'c2']
>>> lst1
['a1', 'b1', 'c1']
>>> lst2
['a2', 'b2', 'c2']
>>> id(lst1)
35957320L
>>> id(lst2)
35873160L
>>> swap(lst1, lst2)
>>> lst1
['a2', 'b2', 'c2']
>>> lst2
['a1', 'b1', 'c1']
>>> id(lst1)
35957320L
>>> id(lst2)
35873160L
Notice that the multiple assignment like a[:] = b[:]
must be used to express copying of the content of the lists.