You need to paint the frame's content to a BufferedImage
first. Try something like...
Container content = frm.getContentPane();
BufferedImage img = new BufferedImage(container.getWidth(), container.getHeight(), BufferedImage.TYPE_INT_RGB);
Graphics2D g2d = img.createGraphics();
content.printAll(g2d);
g2d.dispose();
Once you have that, you can use the ImageIO.write
method, passing the img
to it.
UPDATE
So, I did a really quick test...
I started out with this background image...
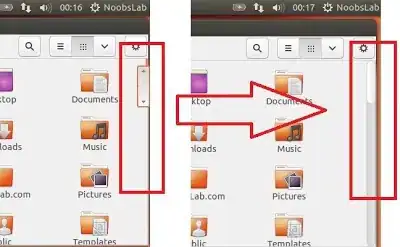
Which I loaded into my frame and laid a JLabel
ontop
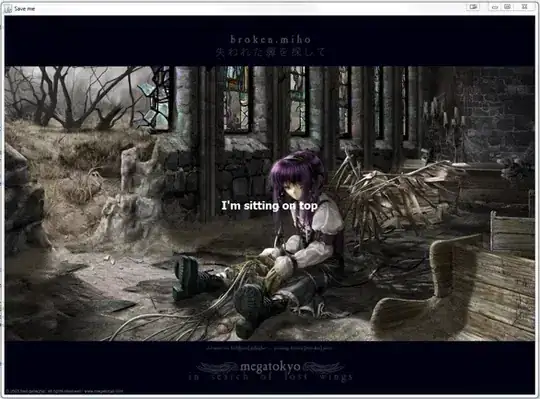
And then saved to a file...
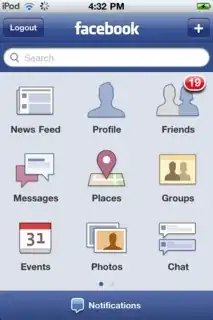
All of which worked fine.
This is the code that I used.
public class TestSaveFrame extends JFrame {
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException ex) {
} catch (InstantiationException ex) {
} catch (IllegalAccessException ex) {
} catch (UnsupportedLookAndFeelException ex) {
}
new TestSaveFrame();
}
});
}
public TestSaveFrame() {
setTitle("Save me");
setDefaultCloseOperation(EXIT_ON_CLOSE);
setLayout(new BorderLayout());
BackgroundPane pane = new BackgroundPane();
pane.setLayout(new GridBagLayout());
JLabel label = new JLabel("I'm sitting on top");
label.setFont(label.getFont().deriveFont(Font.BOLD, 24f));
label.setForeground(Color.WHITE);
pane.add(label);
add(pane);
pack();
setLocationRelativeTo(null);
setVisible(true);
pane.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
if (e.getClickCount() == 2 && e.getButton() == MouseEvent.BUTTON1) {
Container content = getContentPane();
BufferedImage img = new BufferedImage(content.getWidth(), content.getHeight(), BufferedImage.TYPE_INT_RGB);
Graphics2D g2d = img.createGraphics();
content.printAll(g2d);
g2d.dispose();
try {
ImageIO.write(img, "png", new File("C:/PrintMe.png"));
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
});
}
public class BackgroundPane extends JPanel {
private Image background = null;
public BackgroundPane() {
try {
background = ImageIO.read(getClass().getResource("/MT015.jpg"));
} catch (IOException ex) {
ex.printStackTrace();
}
}
@Override
public Dimension getPreferredSize() {
return background == null ? new Dimension(200, 200) : new Dimension(background.getWidth(this), background.getHeight(this));
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
if (background != null) {
int x = (getWidth() - background.getWidth(this)) / 2;
int y = (getHeight() - background.getHeight(this)) / 2;
g.drawImage(background, x, y, this);
}
}
}
}
Without an example of the work flow, it's going to be tough to work out where you're going wrong.
I should note that I use printAll
over paint
because I've had issues with doing this using paint
recently (throwing exceptions and the like)