If you insist on using REGEXTRACT()
to break your path down, you may find the following resources to be helpful:
However, in a Google Spreadsheet, the most effective way to access specific portions of a path & filename string is to use a custom function in an embedded script. I'll assume you're familiar with apps-script programming; see Building Your First Script.
The following code contains two scripts, one built on the other. For example, calling pathExtract()
will return a specified segment of a given path string.
/**
* Split path into parts, return in array. If path started
* at 'root', first part will be "/". This function may be used
* as a custom function in Google Spreadsheets.
*
* @var {string} path The search path, e.g. "/a/b/c/d.txt"
* @return {array} Result of splitting path at "/".
*/
function pathSplit( path ) {
var arr = path.split('/');
if (path[0] === "/") {
arr[0] = "/";
}
return ( arr );
}
/*
* Get a portion of a path string. To match INDEX() function,
* the parameter index is 1-based. Requesting index 0 will
* result in the last element from the string. This function
* may be used as a custom function in Google Spreadsheets.
*
* @var {string} path The search path, e.g. "/a/b/c/d.txt".
* @var {number} index 1-n, the element to extract. 0 for last.
* @return {string} Element [index] from path, or *ERROR*.
*/
function pathExtract( path, index ) {
var result = "*ERROR*";
var pathArray = pathSplit( path );
if (index >= 1 && index <= pathArray.length) {
result = pathArray[index-1];
}
if (index === 0) {
result = pathArray[pathArray.length-1];
}
return ( result );
}
Here's a few examples of what you can do with those scripts added to your spreadsheet:
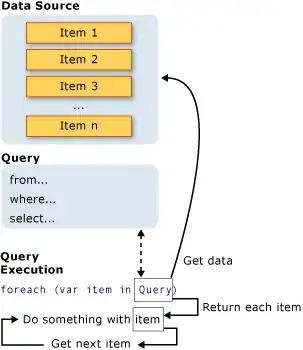
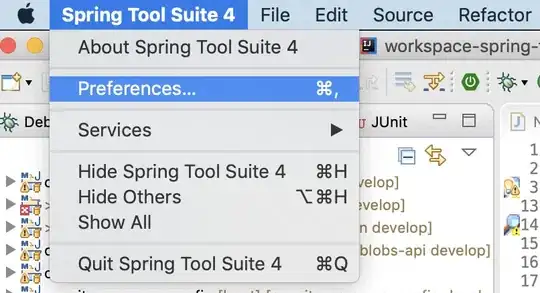