I (think) I see what you are trying to achieve. You are writing your syntax like a mathematical function definition. Matlab is interpreting f
as a 2-dimensional data type and trying to assign the value of the expression to data indexed at x,y
. The values of x
and y
are not integers, so Matlab complains.
If you want to plot the output of the function (we'll call it z
) as a function of x
and y
, you need to define the function quite differently . . .
f = @(x,y)(x-3).^2 - (y-2).^2;
x=2:.2:4;
y=1:.2:3;
z = f( repmat(x(:)',numel(y),1) , repmat(y(:),1,numel(x) ) );
surf(x,y,z);
xlabel('X'); ylabel('Y'); zlabel('Z');
This will give you an output like this . . .
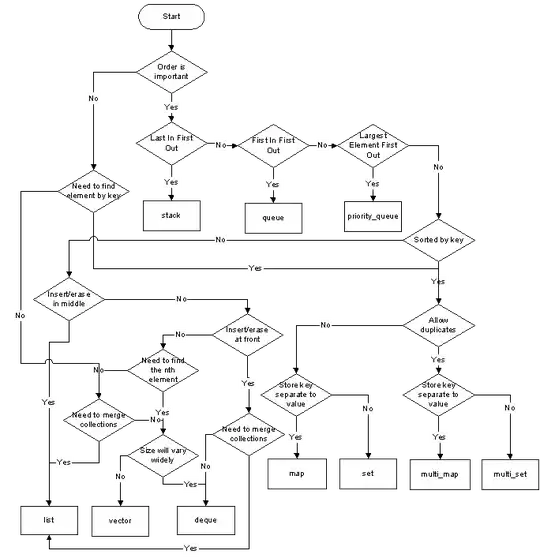
The f = @(x,y)
part of the first line states you want to define a function called f
taking variables x
and y
. The rest of the line is the definition of that function.
If you want to plot z
as a function of both x
and y
, then you need to supply all possible combinations in your range. This is what the line containing the repmat
commands is for.
EDIT
There is a neat Matlab function meshgrid
that can replace the repmat version of the script as suggested by @bas (welcome bas, please scroll to bas' answer and +1 it!) ...
f = @(x,y)(x-3).^2 - (y-2).^2;
x=2:.2:4;
y=1:.2:3;
[X,Y] = meshgrid(x,y);
surf(x,y,f(X,Y));
xlabel('x'); ylabel('y'); zlabel('z');