To add a header that doesn't scroll you could create a controller whose view contains both the additional views you want to add as well as the DialogViewController's view. For example the following simple example adds a UILabel along with the DialogViewController's view as subviews of an additional controller (called container in this case):
[Register ("AppDelegate")]
public partial class AppDelegate : UIApplicationDelegate
{
UIWindow window;
MyDialogViewController dvc;
UIViewController container;
float labelHeight = 30;
public override bool FinishedLaunching (UIApplication app, NSDictionary options)
{
window = new UIWindow (UIScreen.MainScreen.Bounds);
container = new UIViewController ();
container.View.AddSubview (new UILabel (new RectangleF (0, 0, UIScreen.MainScreen.Bounds.Width, labelHeight)){
Text = "my label", BackgroundColor = UIColor.Green});
dvc = new MyDialogViewController (labelHeight);
container.View.AddSubview (dvc.TableView);
window.RootViewController = container;
window.MakeKeyAndVisible ();
return true;
}
}
Then the DialogViewController adjusts the TableView's height in the ViewDidLoad method:
public partial class MyDialogViewController : DialogViewController
{
float labelHeight;
public MyDialogViewController (float labelHeight) : base (UITableViewStyle.Grouped, null)
{
this.labelHeight = labelHeight;
Root = new RootElement ("MyDialogViewController") {
new Section (){
new StringElement ("one"),
new StringElement ("two"),
new StringElement ("three")
}
};
}
public override void ViewDidLoad ()
{
base.ViewDidLoad ();
TableView.Frame = new RectangleF (TableView.Frame.Left, TableView.Frame.Top + labelHeight, TableView.Frame.Width, TableView.Frame.Height - labelHeight);
}
}
Here's a screenshot showing the result in the simulator:
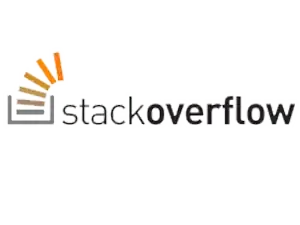