I was a little bit surprised by the results obtained by @pikzen (in 2012), so I performed some test of my own using strtotime()
. I think the problem wasn't thought from the right perspective.
If we are to use strtotime()
with a timestamp, the timestamp should be converted from the start before doing any operations.
E.G. I'm currently writing an algorithm that creates repeated blackouts and holidays over a large period and, of course, needs a lot of date validations and operations.
I ran a little test with 1,000,000 repetitions using PHP 7 and here are my results:
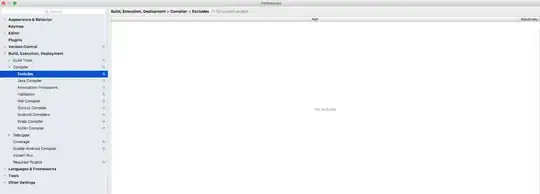
For a complex algorithm that has to deal with a lot of date operations, it seems to me that there's a slight benefit for dealing with integer timestamps rather than string DateTimes.
Here's the code used for my tests:
set_time_limit(180); //3 minutes
echo "<style>table, tr, th, td {border-style:solid; padding:2px;}</style>" .
"<h1>Date add using strtotime() 1,000,000 repetitions</h1>" .
"<table><b><tr style='background-color:LavenderBlush;'><th>Unit</th>" .
"<th>String date with conversion</th><th>String date w/o conversion</th>" .
"<th>Timestamp with conversion</th><th>timestamp w/o conversion</th></tr></b>";
//add day
echo "<tr style='background-color:aqua;'><td><b>Day</b></td>";
$date = date("Y-m-d");
$test = microtime(true);
for ($i = 0; $i < 1000000; $i++) {
$date = date('Y-m-d', strtotime($date . '+1 day'));
}
echo "<td>" . (microtime(true) - $test) . "</td>";
$date = date("Y-m-d");
$test = microtime(true);
for ($i = 0; $i < 1000000; $i++) {
$newDate = strtotime($date . '+1 day');
}
echo "<td>" . (microtime(true) - $test) . "</td>";
$date = strtotime(date("Y-m-d"));
$test = microtime(true);
for ($i = 0; $i < 1000000; $i++) {
$newDate = date('Y-m-d', strtotime('+1 day', $date));
}
echo "<td>" . (microtime(true) - $test) . "</td>";
$date = strtotime(date("Y-m-d"));
$test = microtime(true);
for ($i = 0; $i < 1000000; $i++) {
$date = strtotime('+1 day', $date);
}
echo "<td>" . (microtime(true) - $test) . "</td><tr>";
//add week
echo "<tr style='background-color:LightCyan;'><td><b>Week</b></td>";
$date = date("Y-m-d");
$test = microtime(true);
for ($i = 0; $i < 1000000; $i++) {
$date = date('Y-m-d', strtotime($date . '+1 week'));
}
echo "<td>" . (microtime(true) - $test) . "</td>";
$date = date("Y-m-d");
$test = microtime(true);
for ($i = 0; $i < 1000000; $i++) {
$newDate = strtotime($date . '+1 week');
}
echo "<td>" . (microtime(true) - $test) . "</td>";
$date = strtotime(date("Y-m-d"));
$test = microtime(true);
for ($i = 0; $i < 1000000; $i++) {
$newDate = date('Y-m-d', strtotime('+1 week', $date));
}
echo "<td>" . (microtime(true) - $test) . "</td>";
$date = strtotime(date("Y-m-d"));
$test = microtime(true);
for ($i = 0; $i < 1000000; $i++) {
$date = strtotime('+1 week', $date);
}
echo "<td>" . (microtime(true) - $test) . "</td><tr>";
//add month
echo "<tr style='background-color:LightGreen;'><td><b>Month</b></td>";
$date = date("Y-m-d");
$test = microtime(true);
for ($i = 0; $i < 1000000; $i++) {
$date = date('Y-m-d', strtotime($date . '+1 month'));
}
echo "<td>" . (microtime(true) - $test) . "</td>";
$date = date("Y-m-d");
$test = microtime(true);
for ($i = 0; $i < 1000000; $i++) {
$newDate = strtotime($date . '+1 month');
}
echo "<td>" . (microtime(true) - $test) . "</td>";
$date = strtotime(date("Y-m-d"));
$test = microtime(true);
for ($i = 0; $i < 1000000; $i++) {
$newDate = date('Y-m-d', strtotime('+1 month', $date));
}
echo "<td>" . (microtime(true) - $test) . "</td>";
$date = strtotime(date("Y-m-d"));
$test = microtime(true);
for ($i = 0; $i < 1000000; $i++) {
$date = strtotime('+1 month', $date);
}
echo "<td>" . (microtime(true) - $test) . "</td><tr>";
//add year
echo "<tr style='background-color:LightGoldenRodYellow;'><td><b>Year</b></td>";
$date = date("Y-m-d");
$test = microtime(true);
for ($i = 0; $i < 1000000; $i++) {
$date = date('Y-m-d', strtotime($date . '+1 year'));
}
echo "<td>" . (microtime(true) - $test) . "</td>";
$date = date("Y-m-d");
$test = microtime(true);
for ($i = 0; $i < 1000000; $i++) {
$newDate = strtotime($date . '+1 year');
}
echo "<td>" . (microtime(true) - $test) . "</td>";
$date = strtotime(date("Y-m-d"));
$test = microtime(true);
for ($i = 0; $i < 1000000; $i++) {
$newDate = date('Y-m-d', strtotime('+1 year', $date));
}
echo "<td>" . (microtime(true) - $test) . "</td>";
$date = strtotime(date("Y-m-d"));
$test = microtime(true);
for ($i = 0; $i < 1000000; $i++) {
$date = strtotime('+1 year', $date);
}
echo "<td>" . (microtime(true) - $test) . "</td><tr></table>";
//string date comparation
echo "<br /><h1>Date comparission with 1,000,000 repetition</h1>";
$date = date("Y-m-d");
$i = 0;
$newDate = date('Y-m-d', strtotime($date . '+1 year'));
$test = microtime(true);
while ($i < 1000000) {
if ($date !== $newDate) {
$i++;
}
}
echo "<br / ><b>String date comparission:</b> " . (microtime(true) - $test) . "<br />";
//timestamp comparision
$date = strtotime($date);
$i = 0;
$newDate = strtotime($newDate);
$test = microtime(true);
while ($i < 1000000) {
if ($date !== $newDate) {
$i++;
}
}
echo "<b>timestamp comparission:</b> " . (microtime(true) - $test) . "<br />";