You've defined image
twice...
BufferedImage image = null;
public static void main(String args[]){
BufferedImage image = null;
This essentially means that by the time you get to the paint
method, it is null
as you haven't initialized the instance variable.
Another problem you will have is the fact that you are trying to load the image from a static reference but the image
isn't declared as static
. Better to move this logic into the constructor or instance method.
Don't use JApplet
as your container when you're adding to a JFrame
, you're better of using something like JPanel
. It will help when it comes to adding things to the container.
YOU MUST CALL super.paint(g)
...in fact, DON'T override the paint
method of top level containers like JFrame
or JApplet
. Use something like JPanel
and override the paintComponent
method instead. Top level containers aren't double buffered.
The paint
methods does a lot of important work and it's just easier to use JComponent#paintComponent
... but don't forget to call super.paintComponent
UPDATED
You need to define image
within the context it is going to be used.
Because you declared the image
as an instance field of GraphicsMovement2
, you will require an instance of GraphicsMovement2
in order to reference it.
However, in you main
method, which is static
, you also declared a variable named image
.
The paint
method of GraphicsMovement2
can't see the variable you declared in main
, only the instance field (which is null
).
In order to fix the problem, you need to move the loading of the image into the context of a instance of GraphicsMovement2
, this can be best achived (in your context), but moving the image loading into the constructor of GraphicsMovement2
public GraphicsMovement2() {
try {
File file = new File("C:\\Users/Jonheel/Google Drive/School/10th Grade/AP Computer Science/Junkbin/MegaLogo.png");
ImageInputStream imgInpt = new FileImageInputStream(file);
image = ImageIO.read(file);
}
catch(FileNotFoundException e) {
System.out.println("x");
}
catch(IOException e) {
System.out.println("y");
}
}
The two examples below will produce the same result...
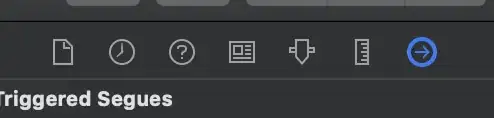
The Easy Way
public class TestPaintImage {
public static void main(String[] args) {
new TestPaintImage();
}
public TestPaintImage() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException ex) {
} catch (InstantiationException ex) {
} catch (IllegalAccessException ex) {
} catch (UnsupportedLookAndFeelException ex) {
}
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new BorderLayout());
frame.add(new ImagePane());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public class ImagePane extends JPanel {
public ImagePane() {
setLayout(new BorderLayout());
ImageIcon icon = null;
try {
icon = new ImageIcon(ImageIO.read(new File("/path/to/your/image")));
} catch (Exception e) {
e.printStackTrace();
}
add(new JLabel(icon));
}
}
}
The Hard Way
public class TestPaintImage {
public static void main(String[] args) {
new TestPaintImage();
}
public TestPaintImage() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException ex) {
} catch (InstantiationException ex) {
} catch (IllegalAccessException ex) {
} catch (UnsupportedLookAndFeelException ex) {
}
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new BorderLayout());
frame.add(new ImagePane());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public class ImagePane extends JPanel {
private BufferedImage background;
public ImagePane() {
try {
background = ImageIO.read(new File("/path/to/your/image"));
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public Dimension getPreferredSize() {
return background == null ? super.getPreferredSize() : new Dimension(background.getWidth(), background.getHeight());
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
if (background != null) {
int x = (getWidth() - background.getWidth()) / 2;
int y = (getHeight() - background.getHeight()) / 2;
g.drawImage(background, x, y, this);
}
}
}
}
Take the time to read through the tutorials