If you would like to get the GUID using a Regex
pattern. Then, try this pattern
(\{){0,1}[0-9a-fA-F]{8}\-[0-9a-fA-F]{4}\-[0-9a-fA-F]{4}\-[0-9a-fA-F]{4}\-[0-9a-fA-F]{12}(\}){0,1}
Example
string findGuid = "hi sdkfj 1481de3f-281e-9902-f98b-31e9e422431f sdfsf 1481de3f-281e-9902-f98b-31e9e422431f"; //Initialize a new string value
MatchCollection guids = Regex.Matches(findGuid, @"(\{){0,1}[0-9a-fA-F]{8}\-[0-9a-fA-F]{4}\-[0-9a-fA-F]{4}\-[0-9a-fA-F]{4}\-[0-9a-fA-F]{12}(\}){0,1}"); //Match all substrings in findGuid
for (int i = 0; i < guids.Count; i++)
{
string Match = guids[i].Value; //Set Match to the value from the match
MessageBox.Show(Match); //Show the value in a messagebox (Not required)
}
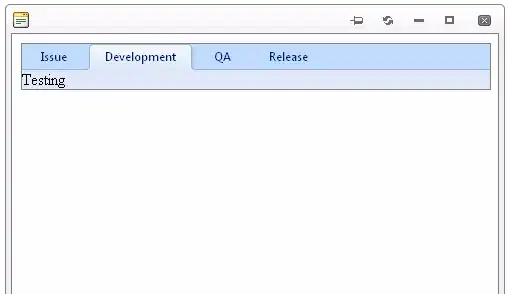
Notice: I've used the same pattern you've provided but simply removed the ^
character which indicates that the expression must match from the start of the string. Then, removed the $
character which indicates that the expression must match from the end of the string.
More information about Regular Expressions can be found here:
Regular Expressions - a Simple User Guide and Tutorial
Thanks,
I hope you find this helpful :)