One other thing you might want to consider is using the Taylor series of the natural logarithm:
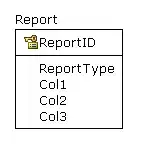
Once you've approximated the natural log using a number of terms from this series, it is easy to change base:
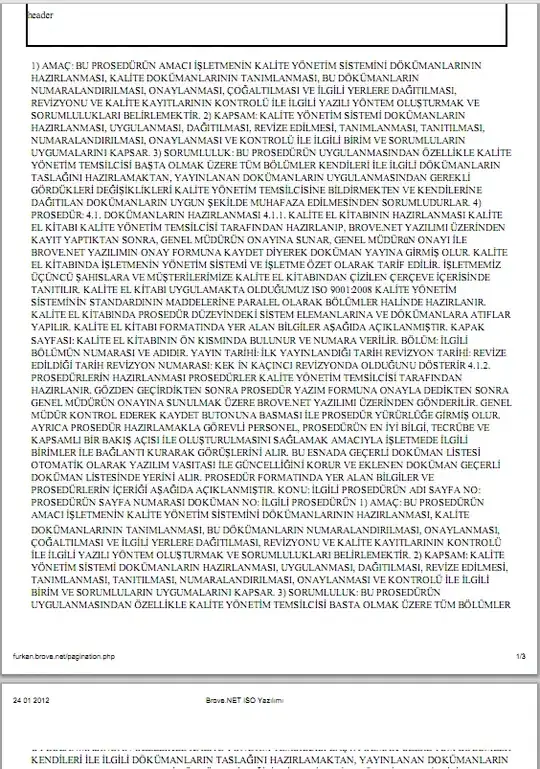
EDIT: Here's another useful identity:

Using this, we could write something along the lines of
def ln(x):
n = 1000.0
return n * ((x ** (1/n)) - 1)
Testing it out, we have:
print ln(math.e), math.log(math.e)
print ln(0.5), math.log(0.5)
print ln(100.0), math.log(100.0)
Output:
1.00050016671 1.0
-0.692907009547 -0.69314718056
4.6157902784 4.60517018599
This shows our value compared to the math.log
value (separated by a space) and, as you can see, we're pretty accurate. You'll probably start to lose some accuracy as you get very large (e.g. ln(10000)
will be about 0.4
greater than it should), but you can always increase n
if you need to.