The following example shows you what you want to do, but with text rather than icon. The program toggles the image, text or whatever you display.
If the reason you used a JLabel is because you wanted a flat button, you can add the code:
private void makeButtonFlat(final JButton makeMeAFlatButton) {
makeMeAFlatButton.setOpaque(false);
makeMeAFlatButton.setFocusPainted(false);
makeMeAFlatButton.setBorderPainted(false);
makeMeAFlatButton.setContentAreaFilled(false);
makeMeAFlatButton.setBorder(BorderFactory.createEmptyBorder());
}
and call this from the
public void makeLayout() {
makeButtonFlat(playButton);
add(playButton);
}
It will then look like a JLabel, but act as a button, which is more logical.
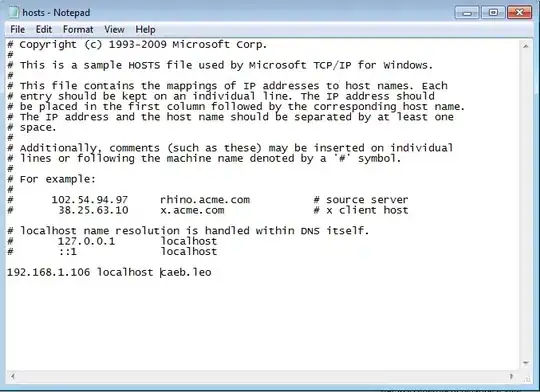
Code snippet:
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
public class Playful {
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
JFrame frame = new JFrame("Playful");
JPanel playPanel = new PlayPanel();
frame.getContentPane().add(playPanel, BorderLayout.CENTER);
frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
frame.setMinimumSize(new Dimension(400, 250));
frame.setLocationRelativeTo(null); // Center
frame.pack();
frame.setVisible(true);
}
});
}
static class PlayPanel extends JPanel {
enum State {
PLAY,
PAUSE;
public State toggle() {
switch (this) {
case PLAY:
return PAUSE;
case PAUSE:
return PLAY;
default:
throw new IllegalStateException("Unknown state " + this);
}
}
}
private State state;
private JButton playButton;
private ActionListener playActionListener;
PlayPanel() {
createComponents();
createHandlers();
registerHandlers();
makeLayout();
initComponent();
}
public void createComponents() {
playButton = new JButton();
}
public void createHandlers() {
playActionListener = new AbstractAction() {
@Override
public void actionPerformed(ActionEvent e) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
toggleState();
}
});
}
};
}
public void registerHandlers() {
playButton.addActionListener(playActionListener);
}
public void makeLayout() {
add(playButton);
}
public void initComponent() {
state = State.PAUSE;
updatePlayButton();
}
private void toggleState() {
state = state.toggle();
updatePlayButton();
}
private void updatePlayButton() {
playButton.setText(state.name());
}
}
}