Create a subclass of UINavigationBar
containing no methods except drawRect:
. Put custom drawing code there if you need to, otherwise leave it empty (but implement it).
Next, set the UINavigationController
's navigation bar to this subclass. Use initWithNavigationBarClass:toolBarClass:
in code, or just change it in the Interface Builder if you're using storyboards/nibs (it's a subclass of your UINavigationController in the hierarchy at the side).
Finally, get a reference to your navigation bar so we can configure it using self.navigationController.navigationBar
in the loadView
of the contained view controller. Set the navigation bar's translucent
to YES
and backgroundColor
to [UIColor clearColor]
. Example below.
//CustomNavigationBar.h
#import <UIKit/UIKit.h>
@interface CustomNavigationBar : UINavigationBar
@end
//CustomNavigationBar.m
#import "CustomNavigationBar.h"
@implementation CustomNavigationBar
- (void)drawRect:(CGRect)rect {}
@end
//Put this in the implementation of the view controller displayed by the navigation controller
- (void)viewDidLoad
{
[super viewDidLoad];
self.navigationController.navigationBar.translucent = YES;
[self navigationController].navigationBar.backgroundColor = [UIColor clearColor];
}
Here's a screen shot of the result, mimicking Plague.
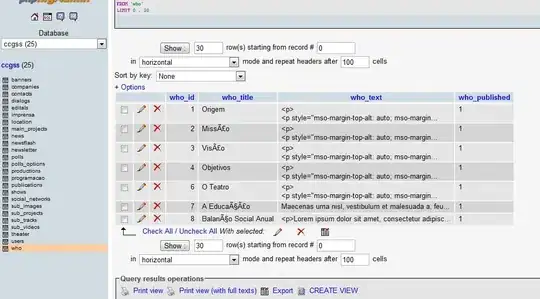
The blue border was drawn in drawRect:
to show you that a UINavigationBar is there and not just a button and a label. I implemented sizeThatFits:
in the subclass to make the bar taller. Both the button and label are UIView's containing the correct UI element that were placed in the bar as UIBarButtonItems. I embedded them in views first so that I could change their vertical alignment (otherwise they "stuck" to the bottom when I implemented sizeThatFits:
).