~
is the bitwise not operator. It inverts the bits of its operand. !
is the logical not operator. The bitwise not operator will return 0
when applied to -1
, which is what indexOf
returns when the value is not found in the array. Since 0
evaluates to false
, doubly negating it will simply return false
(a boolean value, rather than a numeric one):
var index = _sessions.indexOf("_SID_5");
console.log(~index); // 0
console.log(!~index); // true
console.log(!!~index); //false
The bitwise not operator will return a value less than 0 for any other possible value returned by indexOf
. Since any other value will evaluate to true
, it's just a shorthand method (kind of... they are both the same number of characters!) of checking whether an element exists in an array, rather than explicitly comparing with -1
:
if (_sessions.indexOf("_SID_5") > -1) {
// This would work the same way
}
Update
With regards to the performance of this, it appears (in Chrome at least) to be marginally slower than the more common comparison with -1
(which itself is marginally slower than a comparison with 0
).
Here's a test case and here's the results:
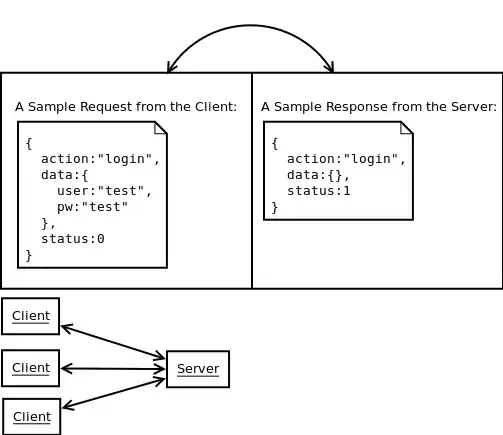
Update 2
In fact, the code in your question can be shortened, which may have been what the author was attempting to do. You can simply remove the !!
, since the ~
will always result in 0
or below (and 0
is the only value that will evaluate to false
):
if (~_sessions.indexOf("_SID_5")) {
// This works too
}
However, in a slightly different situation it could make sense to add in the !
operators. If you were to store the result of the bitwise operator in a variable, it would be a numeric value. By applying the logical not operator, you get a boolean value (and applying it again ensures you get the correct boolean value). If for some reason you require a boolean value over a numeric one, it makes a little bit more sense (but you can still just use the normal comparison with -1
or 0
):
var inArray = !!~_sessions.indexOf("_SID_5");
console.log(typeof inArray); // boolean