It's because the p
variable is shared by your closures, there's just one p variable. By the time your handlers are called, p has changed.
You have to use a technique I call freezing your closures
for (var i = 0; i < that.pairs.length; i++) {
// The extra function call creates a separate closure for each
// iteration of the loop
(function(p){
p.t.click(function() {
that.doToggle(p);
});
})(that.pairs[i]); //passing the variable to freeze, creating a new closure
}
A easier to understand way to accomplish this is the following
function createHandler(that, p) {
return function() {
that.doToggle(p);
}
}
for (var i = 0; i < that.pairs.length; i++) {
var p = that.pairs[i];
// Because we're calling a function that returns the handler
// a new closure is created that keeps the current value of that and p
p.t.click(createHandler(that, p));
}
Closures Optimization
Since there was a lot of talk about what a closure is in the comments, I decided to put up these two screen shots that show that closures get optimized and only the required variables are enclosed
This example http://jsfiddle.net/TnGxJ/2/ shows how only a
is enclosed
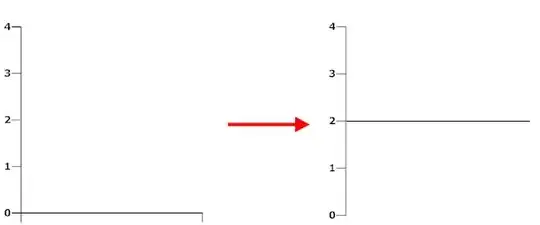
In this example http://jsfiddle.net/TnGxJ/1/, since there's an eval
, all the variables are enclosed.
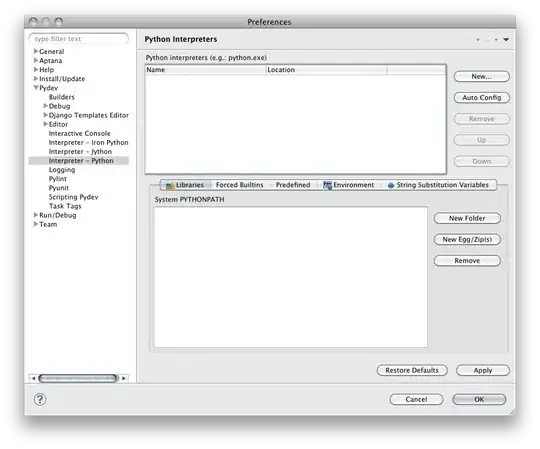