It's possible although there are a bunch of steps which can be easily forgotten. After struggling with myself to get this working for 4 hours I came up with the following solution.
1 - Create a View Controller like any other.
Header file
#import "DDBaseViewController.h"
@interface DDHomeSearchLoadingViewController : UIViewController
@end
Implementation file
#import "DDHomeSearchLoadingViewController.h"
@interface DDHomeSearchLoadingViewController ()
@property (weak, nonatomic) IBOutlet UIActivityIndicatorView *activityMonitor;
@property (weak, nonatomic) IBOutlet UIView *modalView;
@end
@implementation DDHomeSearchLoadingViewController
#pragma mark - UIViewController lifecycle
- (void)viewDidLoad
{
[super viewDidLoad];
[self setupUI];
}
-(void) setupUI
{
[self makeRoundedCorner:self.modalView AndCornerRadius:6.0f];
[self.activityMonitor startAnimating];
}
-(void) makeRoundedCorner:(UIView*) view AndCornerRadius:(float) cornerRadius
{
[view.layer setCornerRadius:cornerRadius];
[view.layer setMasksToBounds:YES];
}
@end
2 - Set your container view background color as ClearColor

3 - Add a UIView which will be presented like an overlay
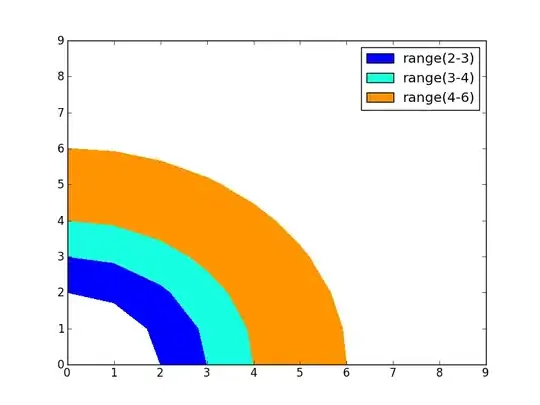
4 - Add a UIView which will be presented like a Dialog Box over the overlay UIView
Make sure it's outside the overlay view when you add/move it. For some reason when you move it using the mouse it adds to overlay UIView automatically. ( Freaking annoying to be honest )
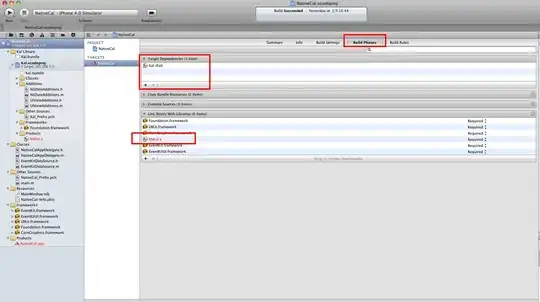
5 - Set the Storyboard ID for the View you've created
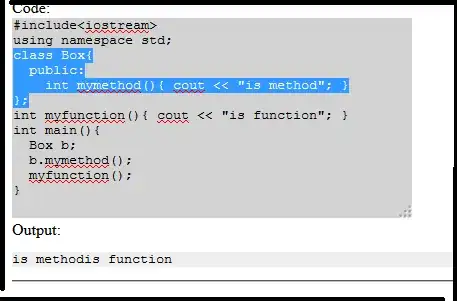
6 - Finally add this piece of code wherever you wan't call it ( Assuming it's a UiViewController too )
DDHomeSearchLoadingViewController* ddHomeSearchLoadingViewController = [[UIStoryboard storyboardWithName:@"Main" bundle:nil] instantiateViewControllerWithIdentifier:@"DDHomeSearchLoadingViewController"];
self.navigationController.modalPresentationStyle = UIModalPresentationCurrentContext;
[self presentViewController:ddHomeSearchLoadingViewController animated:YES completion:nil];
I hope it helps you guys out
Cheers