If you do str(x)
you will see what is going on.
> str(x)
'data.frame': 14 obs. of 3 variables:
$ date : chr "2012-07-27" "2012-10-26" "2012-07-27" "2012-10-26" ...
$ variable: num 30 30 182 182 365 ...
$ value : chr "0.08" "0.12" "0.15" "0.15" ...
value
is a character, not a number as mnel states in his comment. So if you change the value
column to a numeric data type it should at least plot. Whether it gives the output you wish is another issue. The code below seems to work for me.
library(reshape2)
library(ggplot2)
x = structure(list(Series.Description = c("2012-07-27", "2012-10-26"
), `1.month` = c("0.08", "0.12"), `6.month` = c("0.15", "0.15"
), `1.year` = c("0.17", "0.19"), `2.year` = c("0.23", "0.30"),
`5.year` = c("0.59", "0.78"), `10.year` = c("1.47", "1.81"
), `30.year` = c("2.51", "2.94")), .Names = c("Series.Description",
"1.month", "6.month", "1.year", "2.year", "5.year", "10.year",
"30.year"), row.names = c(1L, 4L), class = "data.frame")
# dates as # of days
z=c(30,182,365,730,1825,3650,10950)
names(x)[1]="date"
names(x)[-1]=c(30,182,365,730,1825,3650,10950)
x=melt(x,id.vars=c(1))
x$variable=levels(x$variable)[x$variable]
x$variable=as.numeric(x$variable)
x$value <- as.numeric(x$value)
ggplot(data=x,aes(x=variable,y=value,group=date,linetype=date)) +
geom_line(colour="red") + geom_point(colour="red") +
scale_x_continuous(breaks=z,labels=c("1M","6M","1Y","2Y","3Y","5Y","10Y")) +
scale_linetype_manual(values=c(2,1)) +
scale_y_continuous(limits=c(0,7))
If you actually just want labels at regular intervals along the x-axis, you should probably try changing the scale_x_continuous
to scale_x_discrete
and removing the bit where you fiddle with x$variable
:
x <- structure(list(Series.Description = c("2012-07-27", "2012-10-26"
), `1.month` = c("0.08", "0.12"), `6.month` = c("0.15", "0.15"
), `1.year` = c("0.17", "0.19"), `2.year` = c("0.23", "0.30"),
`5.year` = c("0.59", "0.78"), `10.year` = c("1.47", "1.81"
), `30.year` = c("2.51", "2.94")), .Names = c("Series.Description",
"1.month", "6.month", "1.year", "2.year", "5.year", "10.year",
"30.year"), row.names = c(1L, 4L), class = "data.frame")
# dates as # of days
z <- c(30,182,365,730,1825,3650,10950)
names(x)[1] <- "date"
names(x)[-1] <- c(30,182,365,730,1825,3650,10950)
x <- melt(x, id.vars = c(1))
#x$variable=levels(x$variable)[x$variable]
# x$variable=as.numeric(x$variable)
x$value <- as.numeric(x$value)
ggplot(data = x, aes(x = variable, y = value, group = date, linetype = date)) +
geom_line(colour = "red") + geom_point(colour = "red") +
scale_x_discrete(breaks = z, labels = c("1M","6M","1Y","2Y","3Y","5Y","10Y")) +
scale_linetype_manual(values = c(2,1)) +
scale_y_continuous(limits = c(0,7))
This gives the following output:
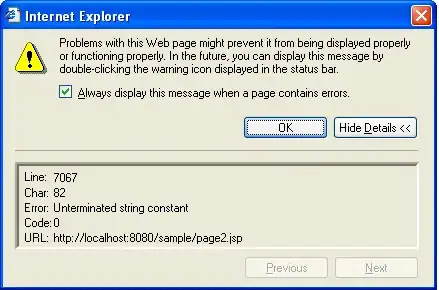
To digress slightly, may I point out that this seems to have been a very simple problem but it hasn't been answered because you made it hard to answer. Why was it hard to answer?
- You didn't include the
library(ggplot)
and other calls at the top.
- You included functions that weren't defined in the initial code.
- You defined structures after the code instead of before.
- You didn't report the error message itself, which would have been useful.
All of this meant that people were copying and pasting into a copy of your code into their R installations and it was failing in multiple ways before they got a chance to see the problem you were experiencing. People here are interested in fixing problems with your code, but they don't want to mess around with issues like the ones outlined above.
On the other hand, if you make it easy for people to help, they will help. So look at your code carefully before posting, copy it, open a new R session, paste it in and see if it works up to the error you have experienced. If it does, great, go ahead and post a question. If not, clean up your code and try again. This is advice from another beginner who has posted a number of not-very-good questions on SO. The fact that you did try to put together a minimal reproducible example suggests that you are keen to get it right, but probably need to do a bit more thinking before posting. This is a great question to revisit.