You want to use a modal dialog of some kind. Dialogs are designed to "halt" execution of the program at the point where they are displayed.
Check out How to Make Dialogs.
You can also use a JOptionPane
to make your life easier, as they can be configured with different buttons/options relatively easily. Check out JOptionPane Features for an overview
Updated with Example
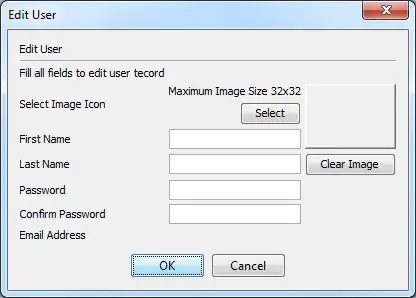
public class TestOptionPane01 {
public static void main(String[] args) {
new TestOptionPane01();
}
public TestOptionPane01() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
}
FormPane formPane = new FormPane();
int result = JOptionPane.showConfirmDialog(
null,
formPane,
"Edit User",
JOptionPane.OK_CANCEL_OPTION,
-1);
switch (result) {
case JOptionPane.OK_OPTION:
System.out.println("You selected okay");
break;
case JOptionPane.OK_CANCEL_OPTION:
System.out.println("You selected cancel");
break;
}
}
});
}
public class FormPane extends JPanel {
public FormPane() {
setLayout(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
gbc.gridx = 0;
gbc.gridy = 0;
gbc.insets = new Insets(2, 2, 2, 2);
gbc.gridwidth = GridBagConstraints.REMAINDER;
gbc.weightx = 1;
gbc.fill = GridBagConstraints.HORIZONTAL;
gbc.anchor = GridBagConstraints.WEST;
add(new JLabel("Edit User"), gbc);
gbc.gridy++;
add(new JSeparator(), gbc);
gbc.gridwidth = 1;
gbc.weightx = 0;
gbc.fill = GridBagConstraints.NONE;
gbc.gridy++;
add(new JLabel("Fill all fields to edit user tecord"), gbc);
gbc.gridy++;
gbc.gridheight = 2;
add(new JLabel("Select Image Icon"), gbc);
gbc.gridheight = 1;
gbc.gridy += 2;
add(new JLabel("First Name"), gbc);
gbc.gridy++;
add(new JLabel("Last Name"), gbc);
gbc.gridy++;
add(new JLabel("Password"), gbc);
gbc.gridy++;
add(new JLabel("Confirm Password"), gbc);
gbc.gridy++;
add(new JLabel("Email Address"), gbc);
gbc.gridx++;
gbc.gridy = 3;
gbc.anchor = GridBagConstraints.EAST;
add(new JLabel("Maximum Image Size 32x32"), gbc);
gbc.gridy++;
add(new JButton("Select"), gbc);
gbc.fill = GridBagConstraints.HORIZONTAL;
gbc.gridy++;
add(new JTextField(10), gbc);
gbc.gridy++;
add(new JTextField(10), gbc);
gbc.gridy++;
add(new JTextField(10), gbc);
gbc.gridy++;
add(new JTextField(10), gbc);
gbc.gridx++;
gbc.gridy = 3;
gbc.anchor = GridBagConstraints.CENTER;
gbc.gridheight = 3;
gbc.fill = GridBagConstraints.BOTH;
JPanel panel = new JPanel();
panel.setBorder(new BevelBorder(BevelBorder.RAISED));
add(panel, gbc);
gbc.gridy += 3;
gbc.gridheight = 1;
gbc.fill = GridBagConstraints.NONE;
add(new JButton("Clear Image"), gbc);
}
}
}