Networkx can do this:
import networkx as nx
def setup_europe():
G = nx.Graph()
G.add_edge("Portugal", "Spain")
G.add_edge("Spain","France")
G.add_edge("France","Belgium")
G.add_edge("France","Germany")
G.add_edge("France","Italy")
G.add_edge("Belgium","Netherlands")
G.add_edge("Germany","Belgium")
G.add_edge("Germany","Netherlands")
G.add_edge("England","Wales")
G.add_edge("England","Scotland")
G.add_edge("Scotland","Wales")
G.add_edge("Switzerland","Austria")
G.add_edge("Switzerland","Germany")
G.add_edge("Switzerland","France")
G.add_edge("Switzerland","Italy")
G.add_edge("Austria","Germany")
G.add_edge("Austria","Italy")
G.add_edge("Austria","Czech Republic")
G.add_edge("Austria","Slovakia")
G.add_edge("Austria","Hungary")
G.add_edge("Denmark","Germany")
G.add_edge("Poland","Czech Republic")
G.add_edge("Poland","Slovakia")
G.add_edge("Poland","Germany")
G.add_edge("Czech Republic","Slovakia")
G.add_edge("Czech Republic","Germany")
G.add_edge("Slovakia","Hungary")
return G
G = setup_europe()
Write a dot file:
nx.write_dot(G, '/tmp/out.dot')
Compute the position of the nodes:
pos = nx.pygraphviz_layout(G, prog = 'dot')
print(pos)
# {'Netherlands': (713.86, 167.0), 'Italy': (473.86, 389.0), 'Czech Republic': (100.86, 241.0), 'Portugal': (879.86, 315.0), 'England': (1024.9, 241.0), 'Denmark': (568.86, 167.0), 'Poland': (100.86, 167.0), 'Scotland': (1024.9, 389.0), 'France': (571.86, 315.0), 'Belgium': (713.86, 19.0), 'Austria': (320.86, 167.0), 'Slovakia': (156.86, 315.0), 'Wales': (990.86, 315.0), 'Switzerland': (473.86, 241.0), 'Hungary': (294.86, 241.0), 'Germany': (465.86, 93.0), 'Spain': (879.86, 241.0)}
Render an png:
agraph = nx.to_agraph(G)
agraph.draw("/tmp/europe.png", format = 'png', prog = 'dot')
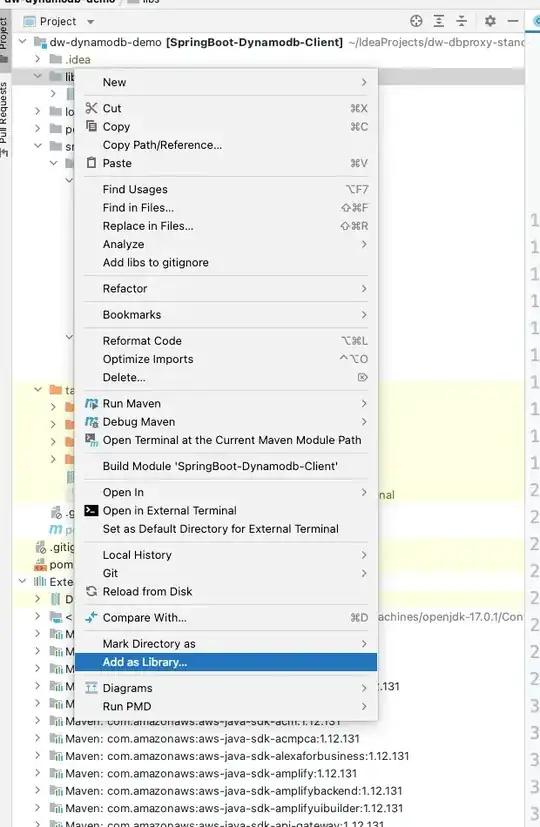