I tried what you asked for DragMouseListener
but found it very uncomfortable so used JInternal Frame
it works fine(but you can still drag images inside JInternal Frame,as I implemented what you asked for).Insted of hand free writing,I used JEditorPane
(you can add hand written thing if you want,but it will be a mess) with accessible parent JScrollPane so that you can type how much you want.
You can drag JInternal Frame easily:
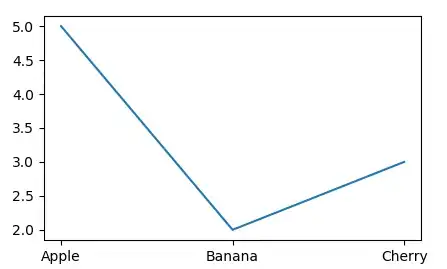
Note: I have used NetBeans GUI Builder for this and suggest you too to use any GUI builder as it makes
typing work easy.
Here is the code:
import java.awt.event.MouseEvent;
public class Move extends javax.swing.JFrame {
public Move() {
initComponents();
}
@SuppressWarnings("unchecked")
private void initComponents() {
jPanel1 = new javax.swing.JPanel();
jInternalFrame1 = new javax.swing.JInternalFrame();
jLabel1 = new javax.swing.JLabel();
jScrollPane1 = new javax.swing.JScrollPane();
jEditorPane1 = new javax.swing.JEditorPane();
jInternalFrame2 = new javax.swing.JInternalFrame();
jLabel2 = new javax.swing.JLabel();
jScrollPane2 = new javax.swing.JScrollPane();
jEditorPane2 = new javax.swing.JEditorPane();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jPanel1.setBackground(new java.awt.Color(0, 0, 0));
jInternalFrame1.setVisible(true);
jLabel1.setIcon(new javax.swing.ImageIcon(getClass().getResource("/images.jpg"))); // NOI18N
jLabel1.setText("jLabel1");
jLabel1.addComponentListener(new java.awt.event.ComponentAdapter() {
public void componentMoved(java.awt.event.ComponentEvent evt) {
jLabel1ComponentMoved(evt);
}
});
jLabel1.addMouseMotionListener(new java.awt.event.MouseMotionAdapter() {
public void mouseDragged(java.awt.event.MouseEvent evt) {
jLabel1MouseDragged(evt);
}
});
jScrollPane1.setViewportView(jEditorPane1);
javax.swing.GroupLayout jInternalFrame1Layout = new javax.swing.GroupLayout(jInternalFrame1.getContentPane());
jInternalFrame1.getContentPane().setLayout(jInternalFrame1Layout);
jInternalFrame1Layout.setHorizontalGroup(
jInternalFrame1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jInternalFrame1Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jInternalFrame1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 203, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel1, 0, 0, Short.MAX_VALUE))
.addContainerGap())
);
jInternalFrame1Layout.setVerticalGroup(
jInternalFrame1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jInternalFrame1Layout.createSequentialGroup()
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 38, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel1, javax.swing.GroupLayout.DEFAULT_SIZE, 250, Short.MAX_VALUE)
.addContainerGap())
);
jInternalFrame2.setVisible(true);
jLabel2.setIcon(new javax.swing.ImageIcon("E:\\untitled.png")); // NOI18N
jLabel2.setText("jLabel2");
jScrollPane2.setViewportView(jEditorPane2);
javax.swing.GroupLayout jInternalFrame2Layout = new javax.swing.GroupLayout(jInternalFrame2.getContentPane());
jInternalFrame2.getContentPane().setLayout(jInternalFrame2Layout);
jInternalFrame2Layout.setHorizontalGroup(
jInternalFrame2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jInternalFrame2Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jInternalFrame2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane2, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.DEFAULT_SIZE, 222, Short.MAX_VALUE)
.addComponent(jLabel2, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.PREFERRED_SIZE, 222, Short.MAX_VALUE))
.addContainerGap())
);
jInternalFrame2Layout.setVerticalGroup(
jInternalFrame2Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jInternalFrame2Layout.createSequentialGroup()
.addContainerGap()
.addComponent(jScrollPane2, javax.swing.GroupLayout.PREFERRED_SIZE, 36, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(jLabel2)
.addContainerGap(40, Short.MAX_VALUE))
);
javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1);
jPanel1.setLayout(jPanel1Layout);
jPanel1Layout.setHorizontalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addContainerGap()
.addComponent(jInternalFrame1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(177, 177, 177)
.addComponent(jInternalFrame2, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(71, Short.MAX_VALUE))
);
jPanel1Layout.setVerticalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jPanel1Layout.createSequentialGroup()
.addGap(49, 49, 49)
.addComponent(jInternalFrame1)
.addGap(209, 209, 209))
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(31, 31, 31)
.addComponent(jInternalFrame2, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(166, Short.MAX_VALUE))
);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jPanel1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
);
pack();
}
private void jLabel1ComponentMoved(java.awt.event.ComponentEvent evt) {
}
private void jLabel1MouseDragged(java.awt.event.MouseEvent evt) {
updateImagePosition(evt);
}
private void updateImagePosition(MouseEvent evt) {
int imageX = evt.getX();
int imageY = evt.getY();
jLabel1.setLocation(imageX, imageY);
}
public static void main(String args[]) {
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new Move().setVisible(true);
}
});
}
private javax.swing.JEditorPane jEditorPane1;
private javax.swing.JEditorPane jEditorPane2;
private javax.swing.JInternalFrame jInternalFrame1;
private javax.swing.JInternalFrame jInternalFrame2;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel2;
private javax.swing.JPanel jPanel1;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JScrollPane jScrollPane2;
}
UPDATE :
See This post answerd by @mKorbel and @trashgod to make JInternal frame minimize/maximize and resize.Great answers.