This line
player = (Player) player.getClass().getInputStream("/sound.wav");
gives you a ClassCastException
because you are trying to cast an InputStream
into a Player
. That's wrong. This line correctly assigns a Player
to your player
variable:
player = Manager.createPlayer(getClass().getInputStream("/sound.wav"), "audio/x-wav");
If you want, you can use my second line again and again, when it's time to replay the sound. You will just be recreating the player. If you are not changing the sound file (.wav), then when the sound finishes playing, I would just start it again without creating a new player:
player.start(); // restart the same sound
But, if you are changing which sound file you use, it might be easier just to call createPlayer
again:
player = Manager.createPlayer(getClass().getInputStream("/another_sound.wav"), "audio/x-wav");
Here are some BlackBerry docs on the Player. Even though you may be on a different J2ME platform, I think the same lifecycle rules probably apply:
A Player has five states: UNREALIZED, REALIZED, PREFETCHED, STARTED,
CLOSED.
The purpose of these life-cycle states is to provide programmatic
control over potentially time-consuming operations. For example, when
a Player is first constructed, it's in the UNREALIZED state.
Transitioned from UNREALIZED to REALIZED, the Player performs the
communication necessary to locate all of the resources it needs to
function (such as communicating with a server or a file system). The
realize method allows an application to initiate this potentially
time-consuming process at an appropriate time.
Typically, a Player moves from the UNREALIZED state to the REALIZED
state, then to the PREFETCHED state, and finally on to the STARTED
state.
A Player stops when it reaches the end of media; or when the stop
method is invoked. When that happens, the Player moves from the
STARTED state back to the PREFETCHED state. It is then ready to repeat
the cycle.
To use a Player, you must set up parameters to manage its movement
through these life-cycle states and then move it through the states
using the Player's state transition methods.
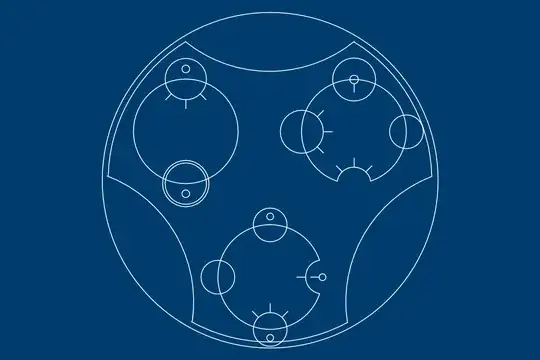
Update: as you seem to be concerned with code complexity, and perhaps deallocating (?) objects, here is a code sample, that hopefully illustrates that it shouldn't be too difficult. You would just take the code in that answer, and make it a method where you pass in the name of the autio file (musicFile
).