You can use NamerFactory
to change information appended to the end of a file. For next test.
[TestFixture]
class Program
{
[Test]
[UseReporter(typeof(WinMergeReporter))]
public void Test1()
{
var image1 = @"firstImage.png";
var image2 = @"secondImage.png";
NamerFactory.AdditionalInformation = Path.GetFileNameWithoutExtension(image1);
ApprovalTests.Approvals.Verify(image1);
NamerFactory.AdditionalInformation = Path.GetFileNameWithoutExtension(image2);
ApprovalTests.Approvals.Verify(image2);
}
}
Approval Tests
has created two files with firstImage
and secondImage
before end. See the screenshot for clarity:
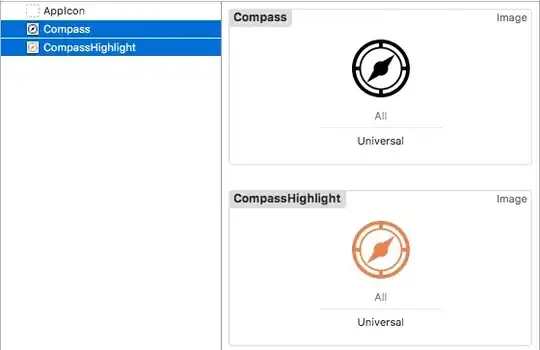
My objects are strings, but for your images all would be the same. You would call Approvals.Verify(image)
as before, but just changing AdditionalInformation
as in the example.
Note: I would not quite recommend verifying two images in one test, because if one Verify will fail - next verifies won't be executed. And there is no way for Approval Tests to concatenate images and verify them in one step, at least if you do this on your own.
Edit: for Java try to use, located at NamerFactory
public static void asMachineSpecificTest(Function0<String> environmentLabeller)
{
additionalInformation = environmentLabeller.call();
}
and provide appropriate Function, that will return names of your images