Wrong data type
You are using the wrong class. You are trying to represent a duration of milliseconds and a time-of-day. Neither fits the Date
class. That class represents a moment (a date, with time-of-day, in context of UTC).
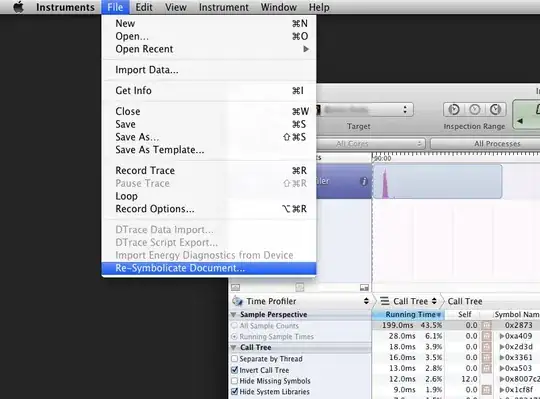
Also, java.util.Date
is a terrible class, designed by people who did not understand date-time handling. Now obsolete.
java.time
The modern solution uses java.time classes.
LocalTime
Specifically, LocalTime
for a time-of-day using a generic 24-hour day, without a date, and without the context of a time zone or offset-from-UTC.
The start of a day for generic days is 00:00:00. We have a constant for that: LocalTime.MIN
. But know that in various time zones, on various dates, the day may start at another time such as 01:00:00.
LocalTime lt = LocalTime.of( 15 , 30 ) ; // 3:30 PM.
Duration
To represent a span-of-time unattached to the timeline, on a scale of hours-minutes-seconds, use Duration
class.
Duration d = Duration.ofMilliseconds( 1_000 ) ;
We can do math with date-time objects.
LocalTime lt = LocalTime.MIN.plus( d ) ;
You should know that java.time classes use a resolution of nanoseconds, much finer than the milliseconds used by the legacy date-time classes.
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.