For non-autolayout storyboards/NIBs, your code is fine. By the way, it's now generally advised that you animate using blocks:
[UIView animateWithDuration:3.0
animations:^{
self.logo.center = CGPointMake(self.logo.center.x, self.logo.center.y - 100.0);
}];
Or, if you want a little more control over options and the like, you can use:
[UIView animateWithDuration:3.0
delay:0.0
options:UIViewAnimationCurveEaseInOut
animations:^{
self.logo.center = CGPointMake(self.logo.center.x, self.logo.center.y - 100);
}
completion:nil];
But your code should work if you're not using autolayout. It's just that the above syntax is preferred for iOS 4 and later.
If you're using autolayout, you (a) create an IBOutlet
for your vertical space constraint (see below), and then (b) you can do something like:
- (void)viewDidAppear:(BOOL)animated {
[super viewDidAppear:animated];
static BOOL logoAlreadyMoved = NO; // or have an instance variable
if (!logoAlreadyMoved)
{
logoAlreadyMoved = YES; // set this first, in case this method is called again
self.imageVerticalSpaceConstraint.constant -= 100.0;
[UIView animateWithDuration:3.0 animations:^{
[self.view layoutIfNeeded];
}];
}
}
To add an IBOutlet
for a constraint, just control-drag from the constraint to your .h in the assistant editor:
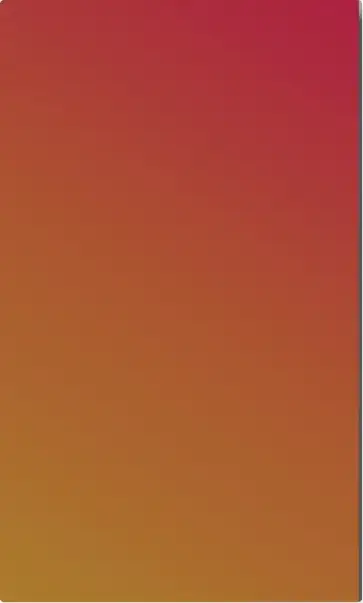
By the way, if you're animating a constraint, be sensitive to any other constraints that you might have linked to that imageview. Often if you put something right below the image, it will have its constraint linked to the image, so you may have to make sure you don't have any other controls with constraints to your image (unless you want them to move, too).
You can tell if you're using autolayout by opening your storyboard or NIB and then selecting the "file inspector" (the first tab on the right most panel, or you can pull it up by pressing option+command+1 (the number "1")):
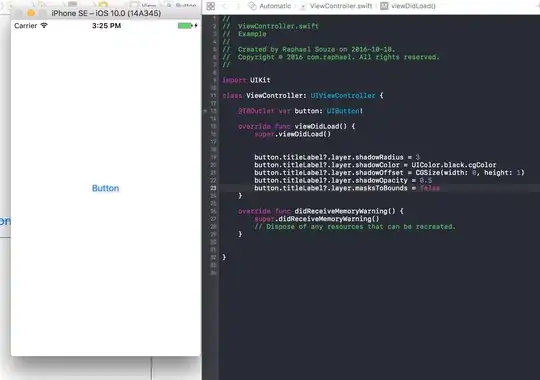
Remember, if you planning on supporting pre-iOS 6, make sure to turn off "autolayout". Autolayout is an iOS 6 feature and won't work on earlier versions of iOS.