You can do this at compile-time by using PostSharp. AOP frameworks which use static code weaving after compilation (also known as the process of postcompilation), provide API for verifying your code design. I've created simple example to demonstrate this.
Define your attribute
public class MyAttribute : Attribute
{
}
Next goes class, to which you want to apply this attribute. I'll comment out attribute to get an error.
//[MyAttribute]
internal class Program
{
private static void Main(string[] args)
{
Console.WriteLine("Hello World");
}
}
Now crate your Aspect
. It will check if any MyAttribute
is present at Program
at postcompile time and if not - populate an error message.
[Serializable]
public class MyValidationAspect : AssemblyLevelAspect
{
public override bool CompileTimeValidate(_Assembly assembly)
{
IEnumerable<object> myAttributes = typeof (Program).GetCustomAttributes(inherit: true)
.Where(atr => atr.GetType() == typeof (MyAttribute));
if (!myAttributes.Any())
Message.Write(MessageLocation.Of(typeof (Program)), SeverityType.Error, "DESIGN1",
"You haven't marked {0} with {1}", typeof (Program), typeof (MyAttribute));
return base.CompileTimeValidate(assembly);
}
}
Then define this aspect on assembly level, like this:
[assembly: MyValidationAspect]
So now, when I try to build solution, I'll get a error:
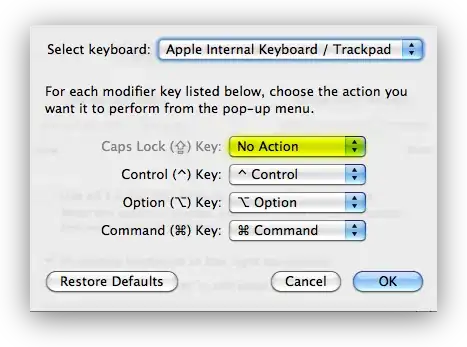
PostSharpPlay.exe
is the name of my console assembly.
If I remove comment before MyAttribute
- the solution compiles and I get no errors.
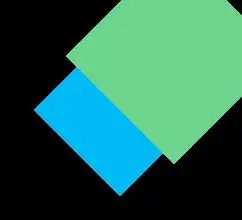