I've done the following test with SQL 2008 R2 and Entity Framework 4.
(The database has the READ_COMMITTED_SNAPSHOT option ON)
I've Created the following Stored Procedure to return the contextual Isolation Level (original from here):
Create Procedure TempTestIsolation
AS
Begin
DECLARE @UserOptions TABLE(SetOption varchar(100), Value varchar(100))
INSERT @UserOptions
EXEC('DBCC USEROPTIONS WITH NO_INFOMSGS')
SELECT Value
FROM @UserOptions
WHERE SetOption = 'isolation level'
End
And then I coded the following test:
static void Main(string[] args)
{
var entities = new MyEntities();
// Execute the SP to get the isolation level
string level = entities.TempTestIsolation().First().Value;
Console.WriteLine("Without a transaction: " + level);
var to = new TransactionOptions() { IsolationLevel = System.Transactions.IsolationLevel.Snapshot };
using (var ts = new TransactionScope(TransactionScopeOption.Required, to))
{
// Execute the SP to get the isolation level
level = entities.TempTestIsolation().First().Value;
Console.WriteLine("With IsolationLevel.Snapshot: " + level);
}
to = new TransactionOptions() { IsolationLevel = System.Transactions.IsolationLevel.ReadCommitted };
using (var ts = new TransactionScope(TransactionScopeOption.Required, to))
{
// Execute the SP to get the isolation level
level = entities.TempTestIsolation().First().Value;
Console.WriteLine("With IsolationLevel.ReadCommitted: " + level);
}
Console.ReadKey();
}
Whose output was:
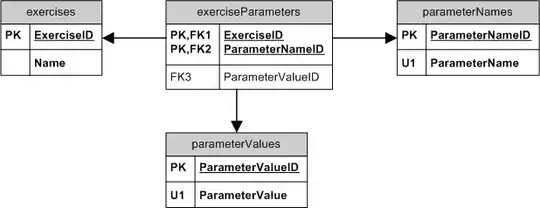
As you can see, when you set the IsolationLevel to Snapshot in your TransactionOptions, the stored procedure executes under the "Snapshot" isolation level, and NOT under "Read Committed Snapshot".
Instead, if you set the IsolationLevel to ReadCommitted it is executed under "Read Committed Snapshot".
Hope it helps.