Simply following the advise in @joran's comment,
DB <- data.frame(
HOUR = c(1, 10, 5, 20),
ID = c(2, 4, 6, 6))
NHOUR <- sprintf("%02d",DB$HOUR) # fix to 2 characters
cbind(NHOUR, DB) # combine old and newdata
NHOUR HOUR ID
1 01 1 2
2 10 10 4
3 05 5 6
4 20 20 6
Update 2013-01-21 23:42:00Z Inspired by daroczig's performance test below, and because I wanted to try out the microbenchmark package, I've updated this question with a small performance test of my own comparing the three different solutions suggested in this thread.
# install.packages(c("microbenchmark", "stringr"), dependencies = TRUE)
require(microbenchmark)
require(stringr)
SPRINTF <- function(x) sprintf("%02d", x)
FORMATC <- function(x) formatC(x, width = 2,flag = 0)
STR_PAD <- function(x) str_pad(x, width=2, side="left", pad="0")
x <- round(runif(1e5)*10)
res <- microbenchmark(SPRINTF(x), STR_PAD(x), FORMATC(x), times = 15)
## Print results:
print(res)
Unit: milliseconds
expr min lq median uq max
1 FORMATC(x) 623.53785 629.69005 638.78667 671.22769 679.8790
2 SPRINTF(x) 34.35783 34.81807 35.04618 35.53696 37.1622
3 STR_PAD(x) 116.54969 118.41944 118.97363 120.05729 163.9664
### Plot results:
boxplot(res)
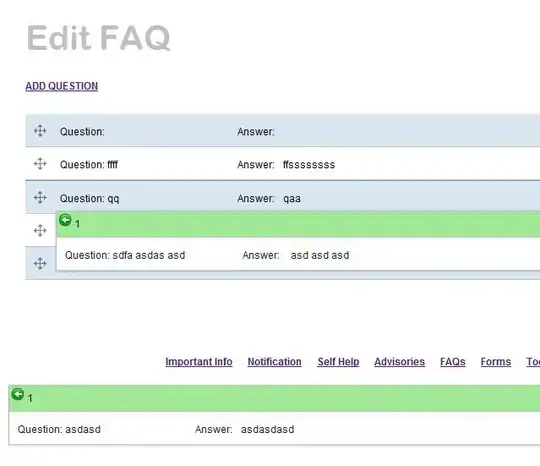