I can't make this script to run all urls from list in one time.
Save your code in a method with one parameter, *args
(or whatever name you wanted, just don't forget the *
). The *
will automatically unpack your list. There is no official name for *
, however, some people (including me) is fond of calling it the splat operator.
def start_download(*args):
for value in args:
##for debugging purposes
##print value
response = urllib2.urlopen(value).read()
##put the rest of your code here
if __name__ == '__main__':
links = ['http://guardsmanbob.com/media/playlist.php?char='+
chr(i) for i in range(97,123)]
start_download(links)
Edit:
Or you could just directly loop over your list of links and download each.
links = ['http://guardsmanbob.com/media/playlist.php?char='+
chr(i) for i in range(97,123)]
for link in links:
response = urllib2.urlopen(link).read()
##put the rest of your code here
Edit 2:
For getting all the links and then saving them in file, here's the entire code with specific comments:
import urllib2
from bs4 import BeautifulSoup, SoupStrainer
links = ['http://guardsmanbob.com/media/playlist.php?char='+
chr(i) for i in range(97,123)]
for link in links:
response = urllib2.urlopen(link).read()
## gets all <a> tags
soup = BeautifulSoup(response, parse_only=SoupStrainer('a'))
## unnecessary link texts to be removed
not_included = ['News', 'FAQ', 'Stream', 'Chat', 'Media',
'League of Legends', 'Forum', 'Latest', 'Wallpapers',
'Links', 'Playlist', 'Sessions', 'BobRadio', 'All',
'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J',
'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T',
'U', 'V', 'W', 'X', 'Y', 'Z', 'Misc', 'Play',
'Learn more about me', 'Chat info', 'Boblights',
'Music Playlist', 'Official Facebook',
'Latest Music Played', 'Muppets - Closing Theme',
'Billy Joel - The River Of Dreams',
'Manic Street Preachers - If You Tolerate This
Your Children Will Be Next',
'The Bravery - An Honest Mistake',
'The Black Keys - Strange Times',
'View whole playlist', 'View latest sessions',
'Referral Link', 'Donate to BoB',
'Guardsman Bob', 'Website template',
'Arcsin']
## create a file named "test.txt"
## write to file and close afterwards
with open("test.txt", 'w') as output:
for hyperlink in soup:
if hyperlink.text:
if hyperlink.text not in not_included:
##print hyperlink.text
output.write("%s\n" % hyperlink.text.encode('utf-8'))
Here's the output saved in test.txt
:
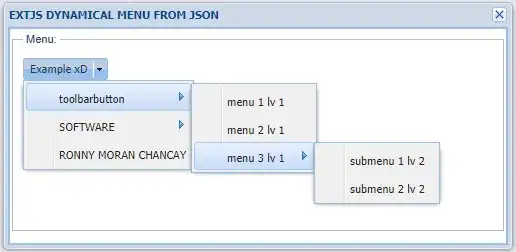
I suggest you change test.txt
into a different filename (ex S Song Titles), everytime you loop your list of links because it overwrites the previous one.