so the library i recommend and use for my code snippet in this answer is not a python library, but it is a python-friendly library, by which i mean that code using this library can be inserted into a python module for processing data and this foreign code will connect to extant python code on both ends, ie, both input and output, and i suspect, although of course i don't know, that's all that's really meant by the "python library" criterion. So if you are writing a web app, this code would be client-side. In other words, this library is not python, but it works with python.
its input is (nearly) raw python dicts, more specifically, json.load(a_python_dict) returns a json array or object, a format which this javascript library can of course recognize; and
the output format is either HTML or SVG, not objects in some
language-specific format
You can use d3.js. It has a class specifically for rendering trees:
var tree = d3.layout.tree().size([h, w]);
There is also a couple of examples of trees (working code) in the example folder in the d3 source, which you can clone/download form the link i provided above.
Because d3 is a javascript library, its native data format is JSON.
The basic structure is a nested dictionary, each dictionary representing a single node with two values, the node's name and its children (stored in an array), keyed to names and children, respectively:
{"name": "a_root_node", "children": ["B", "C"]}
and of course it's simple to convert between python dictionaries and JSON:
>>> d = {"name": 'A', "children": ['B', 'C']}
>>> import json as JSON
>>> dj = JSON.dumps(d)
>>> dj
'{"name": "A", "children": ["B", "C"]}'
here's a python dictionary representation of a larger tree (a dozen or so nodes) which i converted to json as above, and then rendered in d3 as the tree shown in the image below:
tree = {'name': 'root', 'children': [{'name': 'node 2', 'children':
[{'name': 'node 4', 'children': [{'name': 'node 10', 'size': 7500},
{'name': 'node 11', 'size': 12000}]}, {'name': 'node 5', 'children':
[{'name': 'node 12', 'children': [{'name': 'node 16', 'size': 10000},
{'name': 'node 17', 'size': 12000}]}, {'name': 'node 13', 'size': 5000}]}]},
{'name': 'node 3', 'children': [{'name': 'node 6', 'children':
[{'name': 'node 14', 'size': 8000}, {'name': 'node 15', 'size': 9000}]},
{'name': 'node 7', 'children': [{'name': 'node 8', 'size': 10000},
{'name': 'node 9', 'size': 12000}]}]}]}
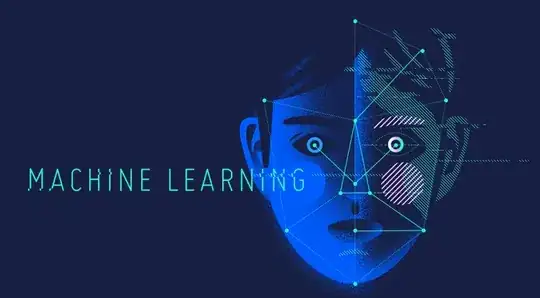
note: d3 renders in the browser; the image above is just a screen shot of my browser window.